Mastering Django: Recipes for Advanced Development
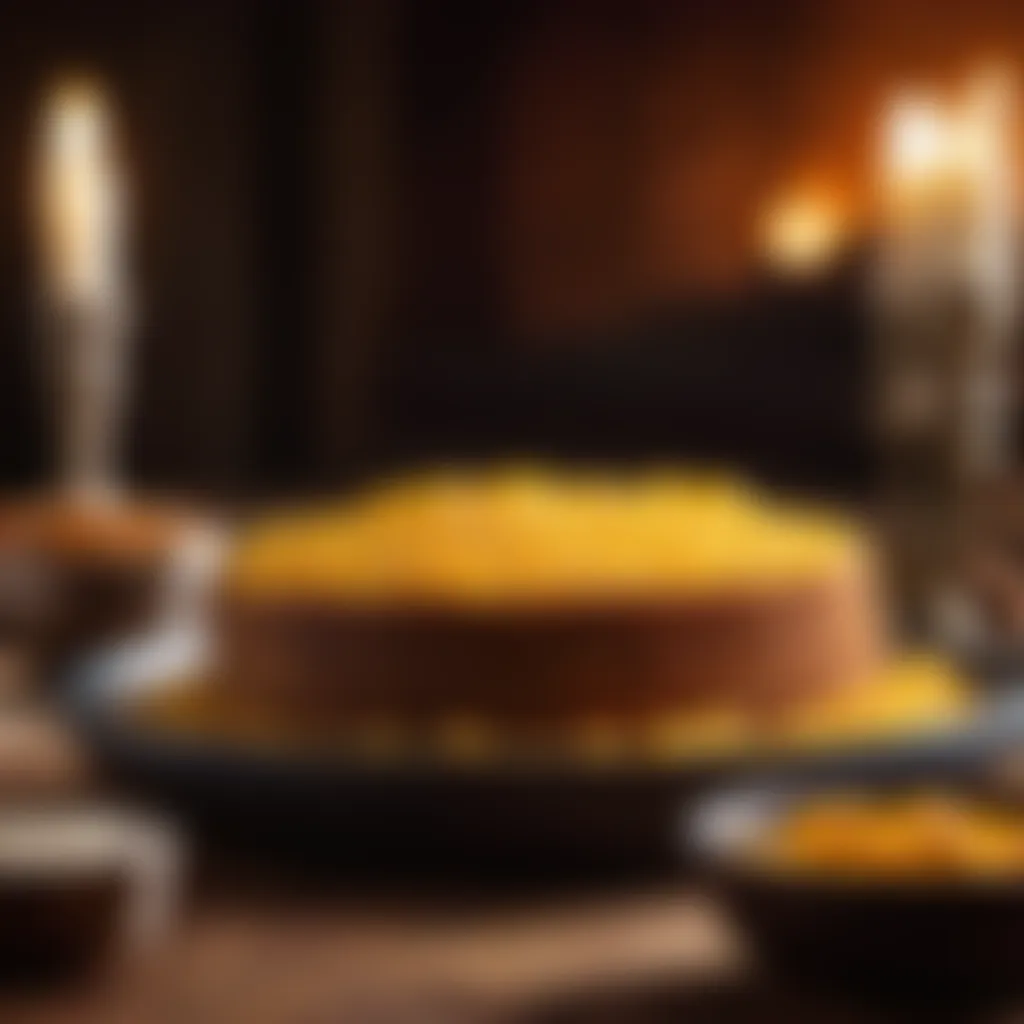
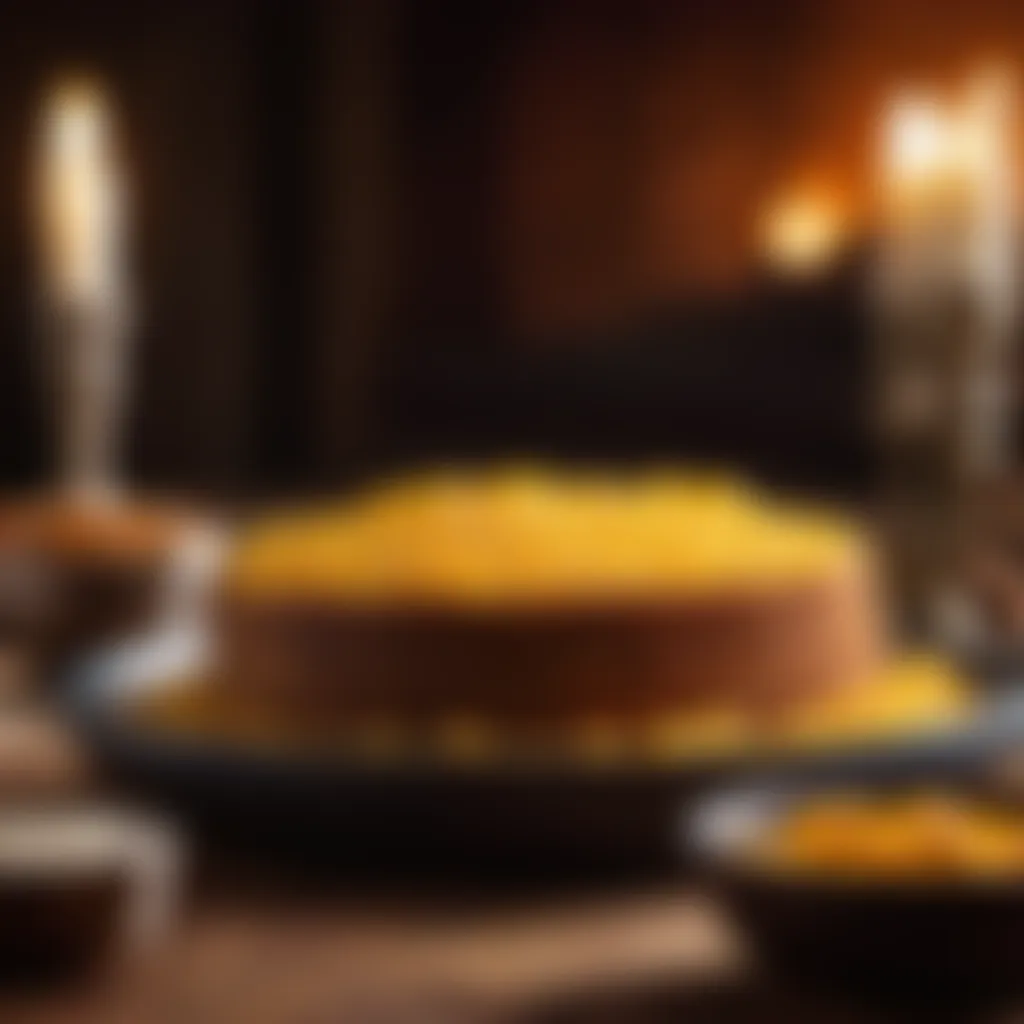
Intro
Django, a powerful framework for web development, has become a staple for developers aiming to deliver high-quality applications efficiently. Think of it as a well-stocked kitchen for programmers, where everything you need is within reach. The flexibility and robustness of Django allow you to whip up an impressive web application with relative ease, much like preparing a gourmet dish with a touch of flair.
In this guide, we dive deep into Django recipes—practical implementations that account for both the basics and the complex intricacies involved in maximizing the framework's capabilities. Whether you're just starting out or are a seasoned pro, this guide offers insights that can help refine your skills. We will address common challenges you might face, much like anticipating the mishaps that can occur when cooking.
By the end of this culinary journey of code, you will not only understand how to whip up a basic Django application but also master advanced techniques that can elevate your projects. So, buckle up as we prepare to dive into the delicious world of Django!
"A well-prepared dish, much like a well-crafted application, requires careful thought, planning, and a hearty dose of creativity."
In the upcoming sections, we'll cover ingredients, preparation steps, technical aspects, and troubleshooting tips to ensure you have everything you'd want to serve an appetizing web app to your users.
Foreword to Django and Its Ecosystem
Django stands as a heavyweight contender in the world of web development frameworks, primarily due to its robust architecture and user-friendly nature. This section lays the groundwork for understanding the intricacies of Django, its components, and the ecosystem that supports it. Knowing this foundational knowledge can aid developers—from novices to seasoned pros—in navigating the complexities that arise during the development process.
Understanding the Django Framework
Django is a high-level Python web framework that promotes rapid development and clean, pragmatic design. What sets it apart from other frameworks is its "batteries-included" philosophy. This means it comes with a multitude of features right out of the box, minimizing the need for third-party tools. The framework is built on the principle of reusability and follows the Don't Repeat Yourself (DRY) philosophy, which advocates for reducing repetition of codes—something essential in efficient programming.
Central to its functionality are its models, views, and templates—often referred to as the MVT architecture. Understanding how these components work harmoniously is vital:
- Models represent the data structure. Think of them as the blueprint of your database. They define the fields and behaviors of the data you’re storing.
- Views determine what data gets displayed and how. They act as the middleman that processes user input, interacts with the models, and renders a response.
- Templates define how the data appears to the user. They determine the layout and design.
Armed with this understanding, developers can start leveraging the full potential of Django tailored to meet the unique needs of their projects. While the syntax may seem daunting at first, once one familiarizes themselves with its structure, they can sail through challenges that arise during implementation.
Historical Context and Evolution
The inception of Django dates back to the early 2000s, developed by a group of web developers who needed a solution for maintaining several websites. With a primary aspiration to create something that allows for efficient development, Django launched its first version in 2005. Since then, its growth trajectory has been impressive, adapting to the rapid technological advancements in web development.
As it evolved, Django incorporated a variety of features that catered to modern web applications, such as:
- Built-in Admin Interface: A lifesaver for developers and designers alike, offering a quick way to manage databases without writing additional code.
- Security Features: With the rise of cyber threats, Django included features like SQL injection protection, cross-site scripting prevention, and more, ensuring developers could create secure applications.
- Community Support: The thriving Django community is indispensable. Through forums like Reddit, developers can connect, troubleshoot, and share best practices, enriching the learning experience.
Django's historical evolution is more than just a timeline; it’s a narrative of continuous improvement. Developers can rest assured that the framework is not just a resource but a living organism, adapting to meet the demands of ever-changing technology landscapes. This adaptability has carved out a niche for Django, establishing it as a reliable choice for new digital projects.
"Just as a chef refines recipes over time, Django matures and integrates the best practices from developers worldwide."
In summary, the introduction to Django and its ecosystem sets the stage for deeper exploration of its core concepts and functionalities. Understanding Django's framework and its historical context allows one to grasp how to exploit its features effectively.
By building a strong foundation here, future sections will seamlessly guide you through everything from setup to deployment.
Core Concepts of Django
Understanding the core concepts of Django is akin to knowing the recipe before starting to cook. This framework is built on a sustainable architecture that helps developers create robust web applications effortlessly. By grasping these essential concepts, you’ll be better equipped to navigate the intricacies of developing with Django. Let’s delve into some fundamental elements that guide the development process.
Models, Views, and Templates Explained
Models, views, and templates are often referred to as the "MTV architecture" of Django, which may sound similar to the classic MVC model but has its own nuances. Think of models as the heart of an application; they represent the data structure. In Django, a model is a Python class that defines the fields, the data types, and any relationships to other models. For example, if you're creating a recipe application, you might have models like , , and , each containing essential attributes such as name, quantity, and skill level.
The views act as the middleman in this setup. They receive user input, manipulate data through the models, and send the corresponding output back to the user, typically through templates. You might think of views as the chef in a restaurant, orchestrating how ingredients (data) are mixed and presented. Each view function processes incoming requests and determines the correct response, integrating data with the right template to serve to the user.
Templates, on the other hand, are where the magic of presentation happens. They dictate how this data is displayed in the user’s browser. By using Django’s template language, developers can create dynamic HTML pages that cater to user requests. This flexibility allows for a cleaner separation of the backend logic and the frontend display, making maintenance smoother and upgrades more efficient.
"A clean separation of concerns is the backbone of efficient web development, enabling teams to innovate without restraint."
In summary, understanding the interplay between models, views, and templates is crucial for anyone looking to maximize the potential of Django. Each component works in tandem, ensuring a cohesive user interaction experience.
Understanding Django Applications
Django applications are essentially the building blocks of your project. Each application is a distinct unit that encapsulates a specific functionality or feature, making your code modular and maintainable. This modular approach offers a significant advantage: you can develop applications independently and integrate them into the larger project as needed.
Imagine you’re crafting a family cookbook. Instead of throwing all recipes into a single untidy mess, you develop separate sections—appetizers, main dishes, desserts—each section being its own application. This organization not only makes it easier to find what you need but also allows for seamless upgrades and tweaks within particular sections without disrupting the entire cookbook.
Moreover, Django's applications can be easily reused across different projects. If you develop a great application for managing recipes, you can use it in future web projects by just swapping it into a different Django project. This capability is a game-changer for productivity, especially when you’re working on multiple projects or scaling existing solutions.
Understanding these concepts is paramount to mastering Django. Grasping how models, views, and templates fit together, and appreciating the modular nature of applications will empower you to become an effective Django developer. From effortlessly managing data to creating intuitive user interfaces, these core elements lay the groundwork for crafting exceptional web applications.
Setting Up Your Django Environment
Setting up your Django environment is the bedrock of your successful web application development. An well-configured environment serves as the crucial launchpad from which you can effectively utilize Django’s capabilities. With the right tools in place, you can focus on building your application without getting bogged down in the technical weeds. A suitable environment also enhances performance, simplifies collaboration, and streamlines deployment processes, ensuring that you can deliver a polished product in due time.
Choosing the Right Development Tools
Selecting the best development tools for your Django project is akin to picking out the right ingredients before embarking on a culinary creation. There are various tools that can set the tone for your entire development experience. Here are some prominent options:
- Visual Studio Code: This lightweight code editor stands out for its flexibility. With its rich ecosystem of extensions catered to Python and Django, you can tailor it to suit your workflow. Features like IntelliSense and integrated terminal enhance speed, making it easier to write and test your code in real time.
- PyCharm: A heavy hitter in IDEs, PyCharm offers a comprehensive set of features for Django development. Its advanced debugging tools, built-in terminal, and code inspection capabilities are designed to streamline your programming process. While it has a steeper learning curve than other editors, the payoff is immense once you’re familiar with its full potential.
- Git: This is not strictly a development tool but essential for any modern development effort. Version control through Git allows you to track changes, experiment with features, and revert back when necessary. Integrating this with platforms such as GitHub opens a world of collaboration and sharing.
- Docker: If you are looking to simplify environment management, Docker allows you to isolate dependencies and ensure your application behaves consistently across development, test, and production phases. Understanding Docker can seem daunting at first, but the benefits often outweigh the initial challenges.
Choosing the right mix might take some trial and error, but ensuring you have a comfortable and efficient setup pays off down the line.
Installing Django and Dependencies
Once you've settled on your development tools, it’s time to get Django and its dependencies up and running. This process is straightforward, yet it does require careful attention to detail. Below is a simple guide to help you through.
- Set up a Virtual Environment: This is an essential first step because it helps isolate your project’s dependencies from the system Python installation. To create a virtual environment, run the following command in your terminal:This command creates a new directory called where your Django installation and any additional packages will live.
- Activate the Virtual Environment: You can activate your new environment by running:You will notice the command line prompt changes, indicating that you're now working within your virtual environment.
- Install Django: With your virtual environment active, you can install Django straight from the Python Package Index using pip:This command fetches the latest version of Django and installs it in your environment.
- Install Additional Dependencies: Depending on your project’s requirements, you may also need to install other libraries. For example, if you plan to interact with a database, you might want to install psycopg2 for PostgreSQL or mysqlclient for MySQL. This can be done the same way:
- On Windows:
- On macOS/Linux:
Remember, your virtual environment allows you the freedom to experiment without fear of breaking things! Feel free to add and remove packages as you explore Django’s wealth of features.
As you set up your environment, keep in mind that a well-prepared foundation is essential for tackling the development challenges ahead. With these steps, you are poised for a smoother journey as you dive into the heart of Django development.
Creating Your First Django Project
Creating your first Django project is like setting the stage for a grand performance. It’s the foundation from which all your web applications will blossom and thrive. Understanding how to effectively structure your project and configure its settings lays the groundwork for smooth sailing in your development journey. Whether you're a seasoned developer returning to Django or a novice eager to explore, this section will guide you through the essential elements and highlights the benefits of thoughtful project creation.
Project Structure Overview
Navigating through Django's project structure is akin to finding your way through a well-organized kitchen. Each component is essential and has its place, making it easier to whip up delightful applications. At the top level, you will find the project folder usually named after your project. Inside, there's a manage.py file, which serves as a command-line tool for interacting with your project. Alongside, there’s the main project directory, featuring your settings, URLs, and WSGI files.
Here’s a quick breakdown of what you'll typically encounter:
- manage.py: Use this to run your project, create applications, and execute migrations.
- settings.py: Contains all your project settings; a pivotal place for configuring your application.
- urls.py: The routing hub where all your URLs come together. Think of it as a menu in a restaurant that directs customers to their desired dish.
- wsgi.py: This file allows your application to communicate with web servers.
Understanding this structure will save you headaches down the line. When you know where to find things, like knowing where to grab your ingredients when cooking, it becomes still easier to implement features and debug issues.
Configuring Settings for Best Practices
Configuring your settings isn’t just about putting together random ingredients; it’s about mixing the right elements to create a well-balanced dish. It's crucial to focus on best practices to enhance your Django project’s security and performance. Here are some key considerations:
- Debugging: Set in production; it’s like not letting your kitchen secrets slip while serving guests.
- Database Settings: Choose robust database backends. SQLite might be fine for testing, but PostgreSQL shines in production with its features and performance.
- Allowed Hosts: Specify allowed hosts with . This acts as a security blanket, ensuring that only specific domains can serve your application.
- Static and Media Files: Configure paths for static assets and user-uploaded media. Managing these paths is vital for keeping your project organized and accessible.
In addition to these settings, familiarize yourself with environment variables to manage sensitive information like secret keys and API tokens. It’s tantamount to putting your fine china away and not letting them collect dust in a busy kitchen.

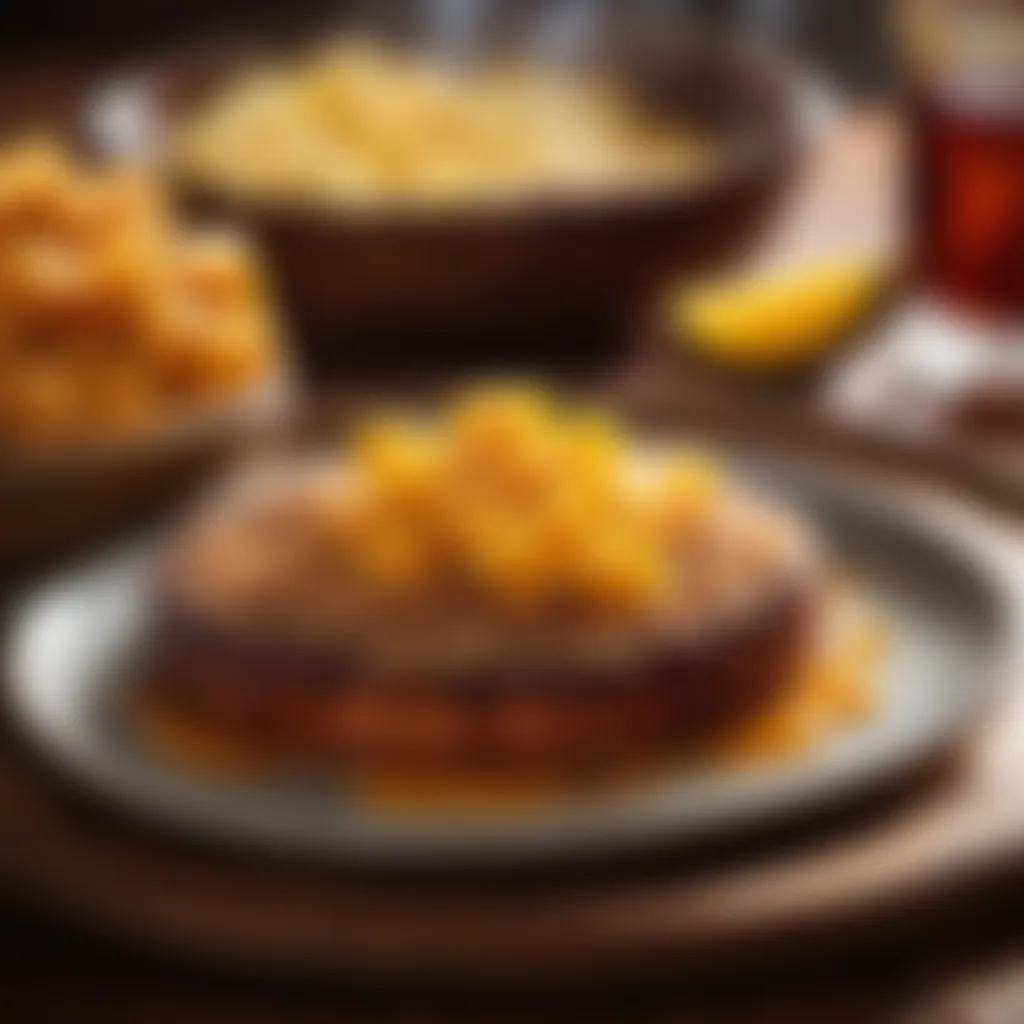
"A well-configured Django project is not just about functionality but also about the ability to evolve and scale over time."
Remember, the foundation you lay during this initial setup will impact every step of your development process. So, take a moment to ensure each setting is thoughtfully configured, and your effort will pay dividends as your project matures.
Effective Database Management in Django
Managing data efficiently is a cornerstone of any web application. In the world of Django, effective database management is not just a technical requirement but also a foundational element that influences the overall performance and scalability of applications. Understanding how to handle databases utilizing Django's capabilities can streamline development, allow for easier maintenance, and ensure data integrity by following best practices.
Django’s built-in ORM (Object-Relational Mapping) system plays a crucial role here. It enables developers to interact with the database using Python code instead of SQL, providing a more intuitive and integrated way to manage data. The use of ORM enhances productivity by simplifying the CRUD (Create, Read, Update, Delete) operations and reducing the probability of errors that might crop up from raw SQL commands.
Using Django ORM for Database Operations
Django ORM serves as the bridge between the Django framework and the underlying database. It allows developers to define their database models as Python classes, which can then be translated to database tables seamlessly. This approach provides several benefits:
- Simplicity: Writing Python code as opposed to SQL can be easier for many developers.
- Abstraction: ORM abstracts away the underlying database complexity, allowing developers to focus purely on application logic.
- Flexibility: With ORM, you can easily switch between different database backends with minimal changes to your code.
To illustrate how the Django ORM works, consider the following example:
In this model, the class represents the desired database table, with attributes corresponding to the columns. Using simple methods, you can create or update instances of this model, leveraging Django’s migration system to reflect changes consistently across the database.
Integrating with External Databases
In many scenarios, it’s essential to connect Django with external databases or data sources. This may arise, for instance, when dealing with legacy systems, or when multiple applications need to share the same data storage. Integrating with external databases comes with its own set of considerations:
- Database Configuration: You need to adjust your file to add connections to additional databases, which might involve specifying multiple database routers.
- Data Integrity: When combining datasets from various sources, you have to be cautious about maintaining data consistency and relationships.
- Performance Considerations: Keep an eye on performance. Data fetching from an external source can introduce latency; optimizing your queries and considering caching might be necessary.
Here's an example of how to set up a connection to an external database in Django:
By integrating with external databases, you expand your application's horizons and enable it to pull information and data across different systems. This can elevate usefulness for end users and open up more sophisticated possibilities in data management.
Taking the time to master effective database management in Django—through the ORM and external integrations—can significantly enhance your application’s capabilities and overall user experience.
"Good database management is not only about performing well but also ensuring data quality and integrity over time."
Whether you're dealing with simple CRUD operations or complex data relationships, mastering these tools will ensure you're equipped for success.
Building RESTful APIs with Django
Building RESTful APIs with Django is not just a trend; it's a necessity in the era of modern web applications. With web and mobile applications increasingly demanding a seamless interaction between client and server, Django provides an efficient way to craft robust APIs. Developers can serve data without getting tangled up in the intricacies of traditional web page rendering. This section delves into why creating RESTful APIs is crucial and looks at the advantages that come with using Django in this domain.
Importance of RESTful APIs in Django
RESTful APIs have revolutionized the way applications communicate, offering a lightweight method to transfer data over HTTP. Here are a few reasons why building RESTful APIs should be a focus for any Django developer:
- Separation of Concerns: It allows front-end and back-end developers to work independently, streamlining the development process.
- Scalability: APIs can easily be scaled as demand increases, making it simpler to handle growing workloads.
- Integration: Different applications can communicate with the API regardless of the technology stack, enabling overall flexibility.
- Easier Maintenance: Changes can be implemented in one part of the system without disrupting the entire application.
The benefits of using Django specifically are noteworthy as it comes packed with features that simplify API creation. Django's robust structure combined with powerful libraries like Django REST Framework makes this task straightforward.
Prolusion to Django REST Framework
The Django REST Framework, or DRF, is a powerful toolkit for building Web APIs. It abstracts many of the complexities involved in developing RESTful services and supplies handy tools that can make life much easier for developers. With DRF, you can easily create serializers, renderers, and more, which facilitate smooth data handling.
- Flexibility: DRF supports various authentication methods, including OAuth and simple token-based systems.
- Serialization: It handles serialization of complex data types, converting them to Python data types usable in APIs.
- ViewSets and Routers: These components allow developers to utilize a simpler approach for managing API views and routes, reducing overhead and improving clarity.
With its extensive documentation and active community, DRF makes diving into RESTful API development not only accessible but also intuitive. Learning how to utilize this framework effectively can save considerable time and reduce the bugs that often plague API development.
Implementing API Endpoints
Setting up API endpoints requires a thoughtful approach. It’s crucial that these endpoints are designed to operate seamlessly, catering to all required applications. Here’s how to implement them effectively:
- Define Your Models: Start with identifying the database models that will serve the API. These models must represent the data structures needed by your application. Ensure they are well-defined to minimize confusion later.
- Create Serializers: Serializers in DRF play a significant role. They convert complex data types into native Python data types that can then be easily rendered into JSON or XML. Keep your serializers simple and aligned with your models.
- Setup Views and ViewSets: Use DRF’s views or viewsets to control how your API processes requests. The viewset allows you to handle multiple requests at once, keeping your code clean and organized.
- Configure URLs: Linking your views with URLs is the final step. Django REST Framework provides a router class that automatically creates the URL routing for viewsets, which helps in reducing boilerplate code.
- Implement Authentication and Permissions: Control access to your API by applying authentication schemes. Whether it be token-based or session-based, decide on your strategy.
Once you've set up your endpoints, it’s key to test them thoroughly. Look out for edge cases and ensure that error handling is in place, giving users clear feedback when issues arise.
Remember: A well-designed API can make or break the user experience. Keep it simple, clear, and functional.
In summation, building RESTful APIs with Django and leveraging the Django REST Framework enables developers to create powerful and maintainable systems that contribute to an overall better application architecture.
Authentication and Authorization Techniques
Authentication and authorization are two foundational pillars in securing a Django application. Without them, user data, private communications, and various functionalities remain vulnerable to exploitation. The beauty of Django lies in its powerful built-in mechanisms to handle these processes, making it essential not just to understand them, but also to implement them effectively in any application.
User Authentication Strategies
Authentication refers to the process by which users provide credentials —typically a username and password—that validate their identity. In the realm of web applications, this segment is crucial for creating personalized and secure experiences for users. A key feature of Django is the built-in authentication framework that offers ready-to-use components, such as user models, forms, and views to facilitate the authentication process.
When deciding on user authentication strategies, it’s important to weigh various methods:
- Session-based Authentication: This traditional method stores user session data on the server. It works well for classic web apps where the server keeps track of users' sessions, allowing for a seamless user experience.
- Token-based Authentication: More suitable for RESTful applications, where stateless interactions prevail. In this approach, the server issues a unique token after the user logs in, and the user sends this token with every subsequent request. The Django REST framework provides robust support for this model.
- Social Authentication: A popular choice for many applications today, social authentication enables users to log in using existing accounts from platforms like Google, Facebook, or Twitter. Libraries like streamline this integration, reducing the friction in user onboarding.
Implementing strong user authentication can drastically reduce unauthorized access, but remember that users’ passwords must be stored securely using techniques like hashing.
Ultimately, a solid authentication strategy not only facilitates user management but also builds trust, as securing their data strengthens user relationships.
Managing Permissions and Roles
Once authentication has validated a user's identity, the next key step is to define what they can and cannot do—this is where roles and permissions come into play. Permissions in Django enable developers to specify access levels on a fine-grained basis, ensuring that each user's experience is tailored accordingly.
Utilizing Django's built-in permission system, you can easily manage these roles:
- User Groups: In Django, users can be organized into groups. By assigning permissions to these groups, you can manage access for multiple users effortlessly. This is particularly useful for large teams where different individuals may require varying levels of access.
- Custom Permissions: Sometimes, out-of-the-box permissions won’t fit all scenarios. Django allows you to create custom permissions, enabling you to define access levels that suit your application's needs precisely.
- Third-party Packages: For projects that require more complex authorization logic, packages like can be invaluable. This library adds per-object permissions, offering a higher level of control over different instances within models.
While managing permissions, it’s essential to adopt a principle of least privilege. That means users should only have access rights necessary to perform their tasks, effectively limiting any potential security risks.
In summary, mastering authentication and authorization in Django not only equips you with a framework to secure your applications but also enhances user experiences by ensuring that access rights are managed efficiently.
Front-End Integration with Django
Front-end integration in Django is crucial as it allows developers to create dynamic web applications that seamlessly blend server-side functionality with user interface elements. This combination ensures that applications are not only powerful behind the scenes but also intuitive and visually appealing for users. When delving into front-end practices within Django, there are several key elements and benefits to consider.
Serving Static Files and Media
One of the cornerstones of web development is the effective management of static files and media content. In Django, static files include JavaScript, CSS, and image files that enhance the user experience and offer visual engagement. Media files, on the other hand, generally pertain to user-uploaded content, such as profile pictures or documents.
- Static File Handling: By default, Django simplifies the serving of static files during development. The built-in development server automatically serves these files from the location. However, during production, one must configure a web server like Nginx or Apache to serve these static assets, ensuring optimized performance.
- Configuration Example: To serve static files in a Django project, you need to define the static URL and root. Here's a simple setup in your file:
- Media Management: Django also provides a built-in system for managing media files.
- Define the media URL and root in your :
Integrating both static and media files effectively makes your application more attractive and user-friendly. By keeping these files organized and served correctly, it enhances the overall performance.
Utilizing JavaScript Frameworks
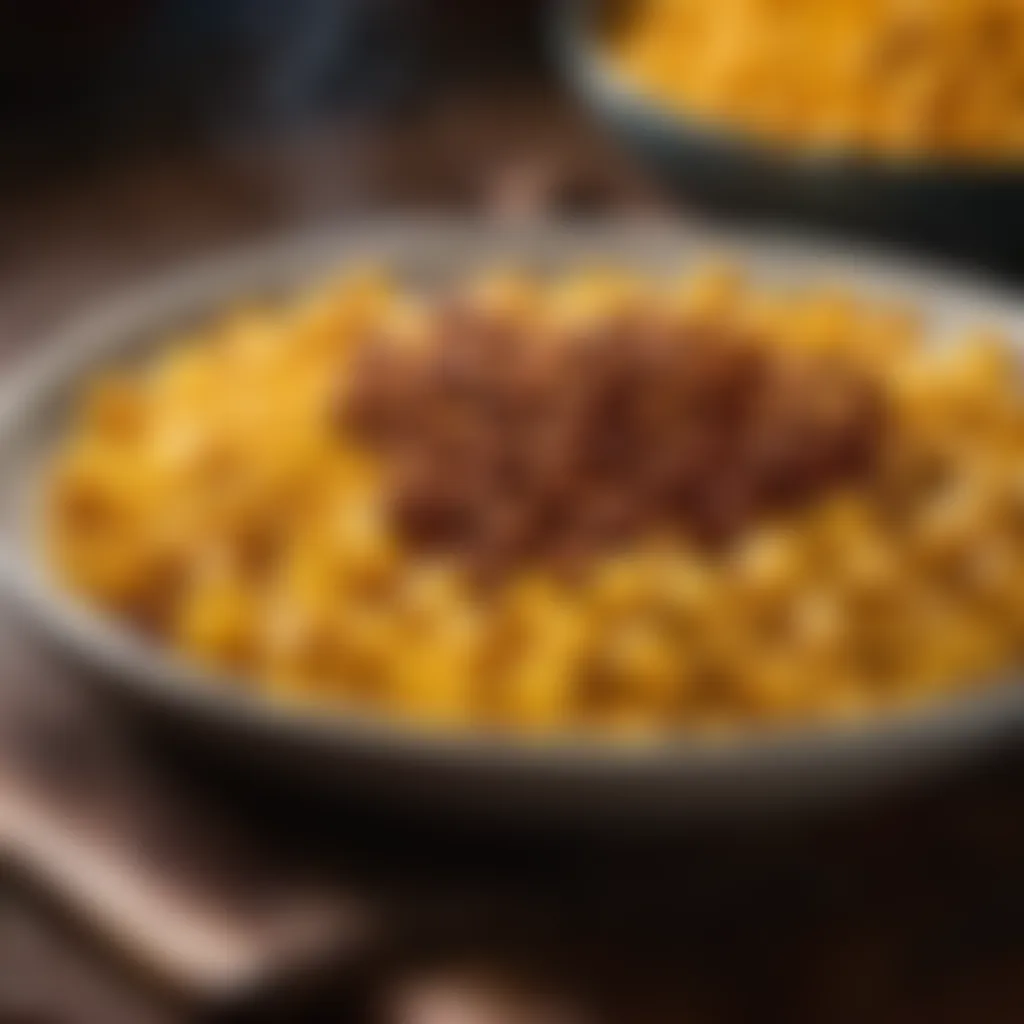
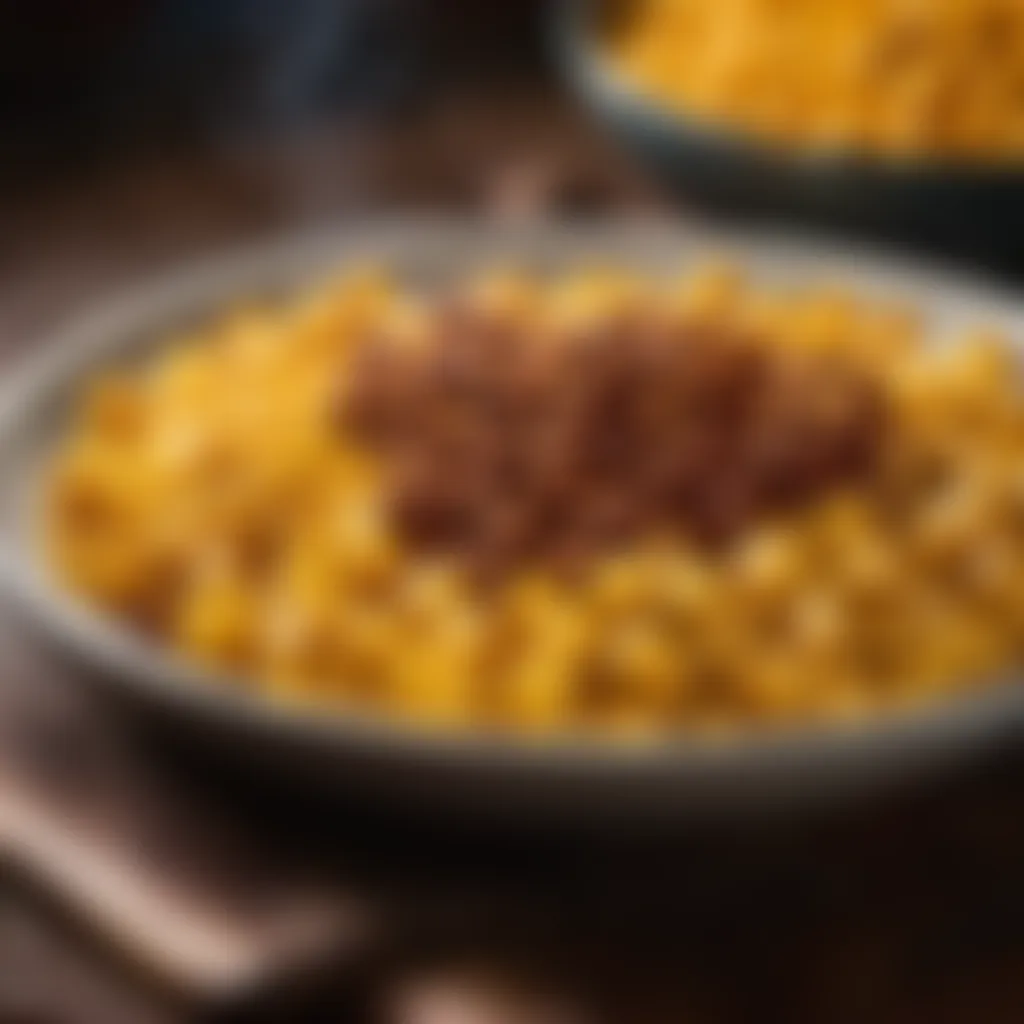
The use of JavaScript frameworks can elevate a Django application. Frameworks like React, Angular, or Vue allow for more responsive and interactive applications. These frameworks enable front-end developers to create single-page applications (SPAs) that interact with Django’s backend through APIs.
- Benefits of JavaScript Frameworks: Integrating these frameworks brings significant advantages:
- Example with React: When integrating React, it can be done through APIs. Django serves the data via its REST API, and React picks it up:
- Improved User Experience: Users can interact with the web application without a whole page reloading, making it smoother and faster.
- Separation of Concerns: By adopting a front-end framework, developers can separate the UI concerns from the server-side logic, leading to cleaner architecture.
- Reusability: Components created can often be reused across projects, speeding up future development.
"The aim is to integrate the dynamic nature of front-end frameworks with the robustness of Django to deliver applications that are not just functional but also enjoyable to use."
Integrating front-end technologies not only elevates the aesthetic value of the web applications but also aligns them with contemporary development practices, crucial for any successful project.
Deploying Django Applications
Deploying a Django application is a vital step that can't be overlooked. It's not just about getting your code out there; it’s about ensuring it runs smoothly, securely, and efficiently in a real-world environment. Think of deployment as turning your home-cooked meal into a dinner party. You want everything to come together perfectly so your guests can savor the fruits of your labor without a hitch. In the world of Django, this means choosing the right hosting conditions, setting up your environment correctly, and maintaining good performance after everything is live.
Evaluating Hosting Options
When it comes to hosting your Django application, the choices are wide and varied. However, not all options are created equally. Evaluating the right hosting service involves considering multiple factors on how it meets the needs of your specific application.
- Shared Hosting: This is great for small, personal projects where traffic is low. It’s cost-effective but can result in slow performance due to shared resources.
- VPS (Virtual Private Server): This gives you more control and is excellent for medium projects requiring additional resources. You can install custom packages that support your project’s needs more closely.
- Dedicated Server: This option is like having a private dining room. You get full control over the server, but it’s usually more expensive and may be overkill for simpler projects.
- Cloud Hosting: Here, scalability is the name of the game. Services like AWS or DigitalOcean allow you to dynamically adjust resources based on traffic, but keep an eye on costs as they can climb rather quickly.
- Platform-as-a-Service (PaaS): Solutions like Heroku simplify deployment, so you’re spending more time cooking than troubleshooting servers. However, pricing may vary, and not all features may be necessary for your particular dish.
By carefully weighing these options, you can find the best host that suits your project’s needs, performance expectations, and budget constraints.
Setting Up Production Environment
Now that you've evaluated your hosting choices, it’s time to roll up your sleeves and set up your production environment. Proper setup can make a world of difference, almost akin to carefully measuring your ingredients before baking a cake.
- Secure Your Application: Start with setting up SSL certificates successfully. It’s important for encrypting traffic between the user and your server, so don’t skip this step. There are providers like Let’s Encrypt that make this task easier.
- Configure Environment Variables: Just like checking your recipe, make sure you’ve got the right ingredients at the ready. Use a file to store sensitive information like your secret keys and database credentials.
- Static and Media Files: Configure your application to serve static files efficiently. You can use services like Amazon S3 or equivalent to store and serve media content, ensuring quicker loading times for your users.
- Database Setup: Ensure your database is properly migrated and optimized for production. Running makes certain all changes are applied so your data stays organized.
- Setting Debug to False: When deploying in production, it’s crucial to turn off debug mode to prevent sensitive information from leaking.
- Monitoring and Logging: Like having a kitchen timer, set up monitoring tools to keep track of your application’s health and performance. Look for platforms that provide logs and alerts to inform you of any issues.
Deploying may seem complicated, but taking each step thoughtfully lessens the risks and opens a pathway for smoother operations.
Putting this all together ensures that your Django application is not only deployed but primed to thrive in a busy kitchen of the web. With a solid deployment strategy, you can focus more on refining your recipe (code) rather than worrying about the oven temperature (server performance). Ultimately, a well-deployed Django project translates to a better experience for users, paving the way for the success of your web journey.
Testing in Django
Testing is often an overlooked step in the software development process, but in Django, it is a vital cog in ensuring application reliability and performance. Why is testing important? Think of it like baking a cake; if you don’t check the oven timer and the cake overspills, you might end up with a soggy mess. In the same way, without thorough testing, your Django application can become riddled with bugs that rear their ugly heads at the worst possible moment.
The main benefit of testing in Django lies in its ability to catch issues early in development. Each minute bug that goes unnoticed can snowball into larger problems down the line, creating a cycle of frustration. Also, as a developer, knowing that your code has been tested gives you confidence. When you change a feature or update the application, running tests ensures that nothing has broken unexpectedly.
Unit Testing Your Django Code
Unit testing focuses on testing individual components of your code in isolation. In a Django project, this means writing tests for your models, views, and forms. Django provides a built-in testing framework, leveraging Python’s module, which makes the process pretty straightforward.
To start unit testing in Django, you can create a test file alongside your model or view files. Here’s a simple way to create a test:
This test checks if your model returns the expected string representation when called. It’s a small step, but it’s crucial. By asserting the conditions of your model, you lock down its functionality.
Make a habit of writing tests for every new feature you implement. This approach will save you a lot of headaches down the line.
Integration Tests and Best Practices
While unit tests focus on individual components, integration tests assess how different parts of your application work together. For instance, suppose you have a view that pulls data from your model. Integration tests would check if the view returns the correct data when accessed.
Here’s a brief rundown of best practices for integration testing in Django:
- Use Fixtures: Fixtures are a great way to set up the initial data for your tests. This approach ensures each test has the same starting point.
- Keep Tests Autonomous: Don’t rely on one test passing or failing based on another test's results. Each test should be independent.
- Be Descriptive: Name your tests so that others (or you, later) can easily understand what each test is meant to verify.
"Testing is not an afterthought; it is an integral part of the development process."
With integration testing, you may find yourself using Django's client methods to simulate requests and check responses. Here’s an example of what that might look like:
This strategy ensures that you are verifying not just the individual pieces but how they function together as well. Both unit and integration testing aim for the same goal: robust and reliable code.
A well-tested Django application will not only enhance user experience but will also make your life as a developer significantly easier. You’ll be able to embrace changes with confidence, knowing that you’ve got your bases covered.
Handling Common Challenges in Django Development
When diving into Django development, encountering challenges is like finding unexpected lumps in a cake batter—disheartening but totally normal. Understanding how to tackle these challenges can actually enhance your skills and deepen your grasp of the framework. In this article section, we’ll discuss specific elements that contribute to successfully navigating the obstacles that may arise when building applications with Django.
Debugging Techniques and Tools
Debugging can feel like trying to pin the tail on a moving donkey. Whichever way you seem to turn, the issues persist. This is why utilizing effective debugging techniques and tools is crucial.
- Debug Toolbar: This is a must-have for any Django developer. The Django Debug Toolbar can assist in revealing overhead, making it easy to spot slow SQL queries or template rendering times. Simply install it via pip and add it to your installed apps. It works wonders in a development environment.
- Logging: There’s a saying that goes, "An ounce of prevention is worth a pound of cure." Proper logging can save you a heap of trouble in the long run. By configuring the Django logging settings, you can track errors, warnings, and general application behavior.
- Unit Tests: Writing unit tests may seem like an additional chore, however, they are indispensable for catching bugs early. With Django’s built-in testing framework, you can ensure that each component functions as expected before introducing them into production.
To give you a clearer picture, here’s a brief code snippet demonstrating how to create a simple test case:
Using these techniques effectively can ensure that you spend more time coding and less time chasing after bugs.
Performance Optimization Strategies
Performance matters like the icing on a cake— without it, even the best recipes can fall flat. Optimizing performance in Django applications is vital to delivering a seamless user experience. Here are several strategies to consider:
- Database Query Optimization: Minimize the number of queries your app makes. Use select_related and prefetch_related to reduce redundancy. Believe me, this can significantly improve response time by limiting the number of database hits.
- Caching: Caching is akin to storing leftover cake for later; why bake again when you already have a slice? Leverage Django’s caching framework with options like file-based, memory, or even caching at the database level to speed up data retrieval.
- Static File Handling: Serving static files can slow down your application if not arranged properly. Use the Django storage framework or a dedicated service like Amazon S3 for better load management. This ensures your web content doesn’t get bogged down in traffic.
Keep in mind that performance tuning is an ongoing effort. Always analyze your application's performance using tools like New Relic or Google PageSpeed Insights.
Advanced Features of Django
In the landscape of web development, it is crucial to not only work with the basics, but to also seek out the more advanced aspects of a framework. Django, with its rich ecosystem, provides numerous advanced features that can greatly enhance an application’s functionality. Understanding these elements helps in making informed decisions as you build and maintain your projects. Here we will delve into two key features: middleware and signals, both of which are instrumental in elevating the Django experience.
Exploring Middleware Capabilities
Middleware in Django operates as a framework for processing requests globally before they reach the view or, conversely, in processing responses before they are sent to the client. Think of middleware as a layer of the cake, sitting between the server and your Django application, adding flavors and textures that are crucial for the overall development experience.
The importance of middleware lies in its ability to simplify and manage various aspects of request and response handling. For instance, it can be used to implement features such as user authentication, session management, and even cross-site request forgery protection. Here are a few key aspects to consider:
- Modular Design: Middleware components can be added or removed easily, promoting a clean and organized codebase.
- Reusable Logic: Common functionality can be packaged into middleware, reducing redundancy across your views and enhancing maintainability.
- Performance Insights: Through logging middleware, you can gain insights into your application’s performance, identifying potential bottlenecks.
To illustrate, consider a scenario where you want to log all incoming requests. You might create a custom middleware like this:
This snippet serves as a fundamental way to track the requests flowing through your application. By capturing this information, you can make better-informed performance optimizations later on.
Using Signals to Enhance Functionality
Django’s signal feature allows different parts of an application to communicate or notify each other when certain actions occur. This is particularly useful for decoupling components, enabling you to maintain a clear separation of concerns. Imagine you're baking a cake, and you want your icing to only be applied once the cake is fully baked; this is the role of signals.
Why are signals beneficial? Here are a few important considerations:
- Decoupling Logic: Events can trigger actions without components being tightly interconnected.
- Flexibility: Signals can enhance functionality by allowing new features to be added without modifying existing code.
- Event-Driven Architecture: This approach opens up the possibility for reactive programming, where changes in one component lead to automatic responses in another.
A simple example of a signal could be a user profile that needs to be created whenever a new user registers. Here’s how you might implement it:
This code listens for the signal from the model, creating a new profile when a user is registered. Such intricate functionalities showcase the power and flexibility of Django’s signals.
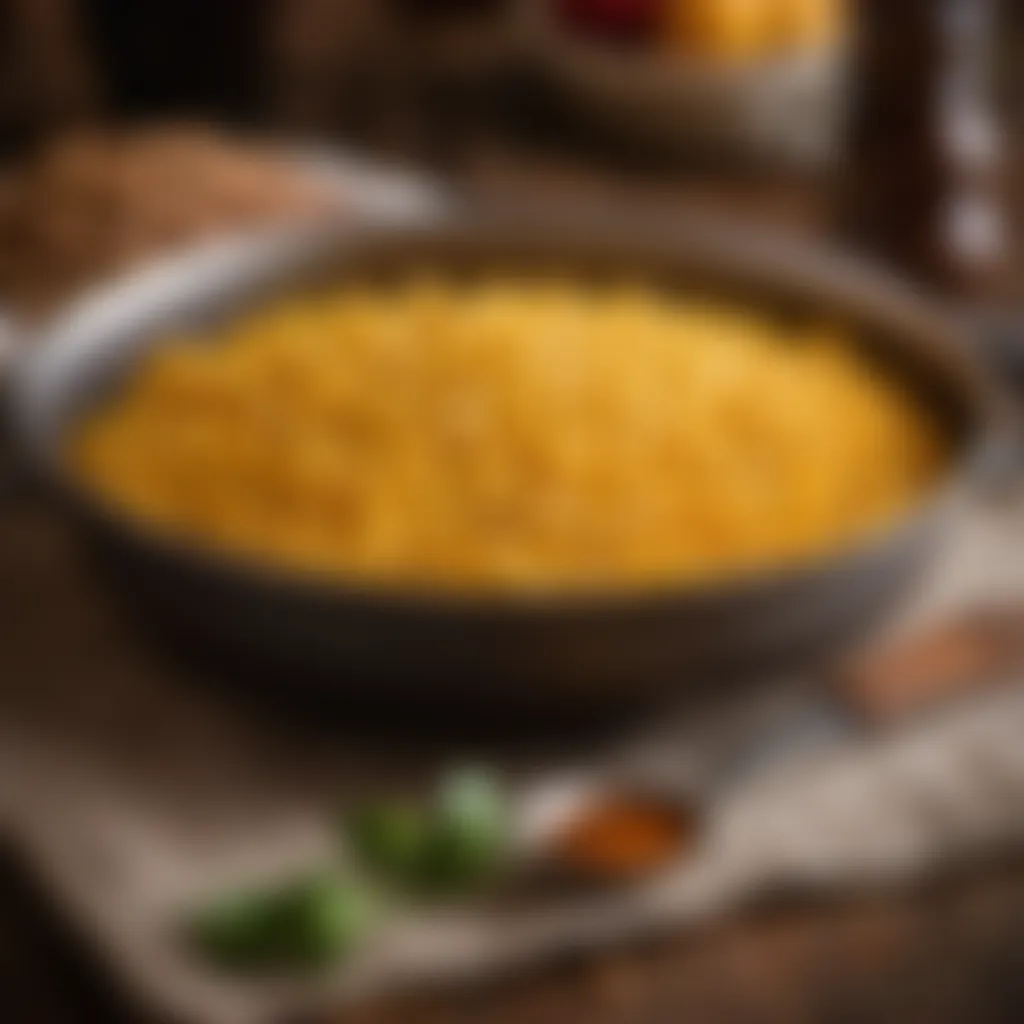
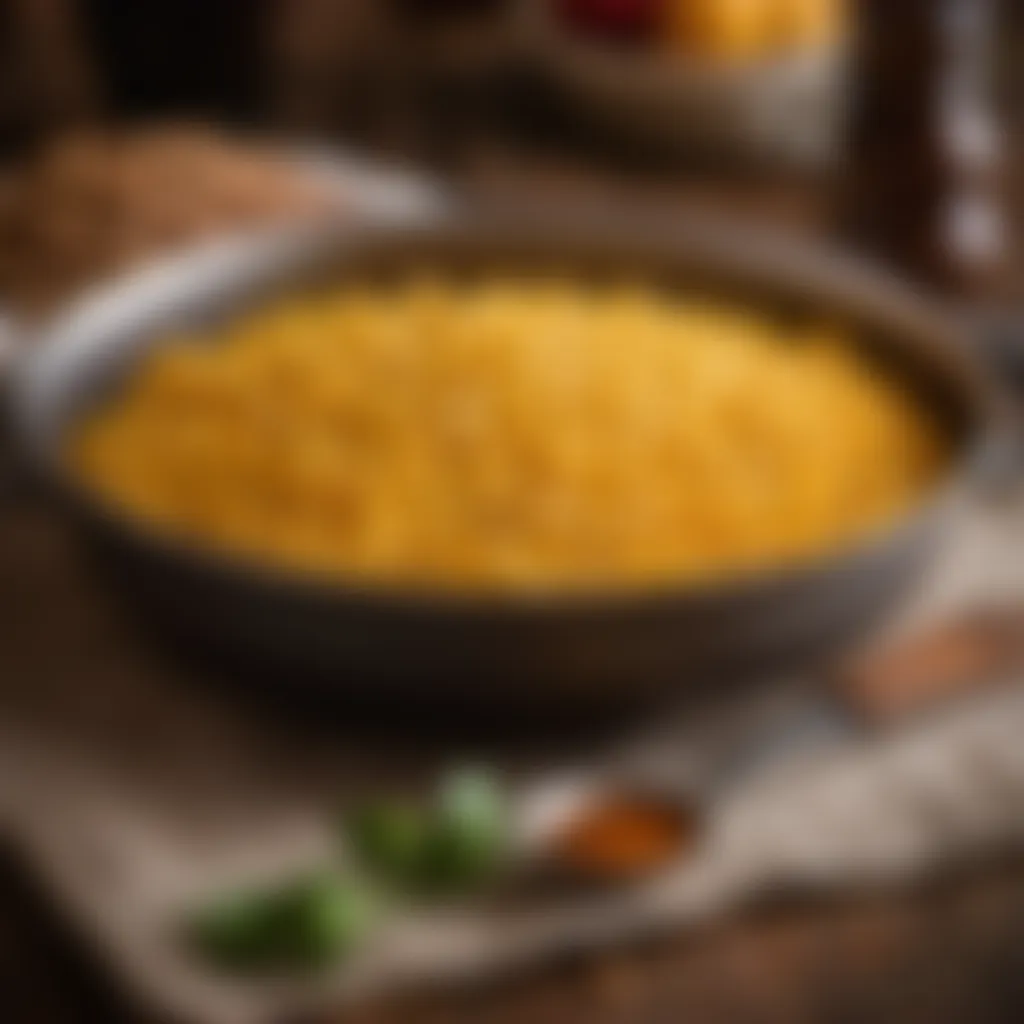
"Understanding advanced features like middleware and signals can significantly enhance your Django applications, making them more efficient and easier to maintain."
Extending Django with Packages
Django, at its core, is a robust framework that provides a solid foundation for web development. However, one of the most powerful features it offers is its extensibility through packages. Extending Django with packages allows developers to leverage pre-built tools, boosting productivity and enhancing functionality without having to reinvent the wheel.
The Importance of Extending Django with Packages
Adding packages not only simplifies the coding process but also enriches a developer's toolkit. With a plethora of third-party packages available, essentials such as authentication, form handling, and data visualization can be implemented easily. This community-driven ecosystem encourages collaboration and accelerates the pace of development.
Moreover, using well-maintained packages can save time while ensuring security compliance. Packages are frequently updated, meaning they might address new vulnerabilities or adapt to changes in related technologies. Hence, developers can stay focused on their application's unique features instead of getting bogged down by routine tasks.
Benefits of Using Django Packages
- Time Efficiency: Implement complex features with just a few lines of code.
- Best Practices: Benefit from peer-reviewed solutions that mirror industry standards.
- Community Support: Gain camaraderie with other developers who use the same tools.
- Easier Maintenance: Third-party packages often receive regular updates and improvements, ensuring code reliability.
Considerations When Choosing Packages
When integrating packages, it’s wise to vet them properly. Not all packages are created equal, so checking their documentation, recent activity, and community ratings can be invaluable. Moreover, consider whether the package aligns with your project's long-term goals or if it simply solves a problem in the short term.
Popular Third-Party Packages for Development
Among the many packages that Django offers, a few stand out as particularly useful:
- Django Rest Framework: Perfect for building Web APIs efficiently, it simplifies the entire process of developing RESTful services.
- Django Allauth: This package tackles authentication seamlessly, supporting various authorization methods, from social logins to email sign-ups.
- Django Celery Beat: If your project requires periodic background tasks, Celery is a fantastic choice, handling task scheduling neatly.
- Django Crispy Forms: This tool enhances the user experience by providing an elegant way to manage forms and layout rendering.
These popular choices reflect a good blend of utility, community support, and active maintenance.
Creating Your Own Django Packages
Sometimes you might find that existing packages don’t quite meet your needs. In these situations, creating your own Django package can be an exciting journey.
Steps to Create Your Own Package
- Define The Purpose: Start by clarifying what your package will do. Make sure there’s a clear need.
- Structure Your Package: Organize your files to fit Django’s conventions, ensuring easy integration.
- Write Code: Focus on writing clean, modular code that is easy to understand and maintain.
- Document Extensively: Good documentation is essential. It enhances user experience and aids in future maintenance.
- Test, Test, Test: Ensure your package works flawlessly before you consider sharing it with others. Use Django’s built-in testing tools to help.
- Share with the Community: If you feel your package is valuable, consider sharing it on platforms such as GitHub or the Python Package Index.
Creating robust Django packages is both a challenging and rewarding endeavor. Through this, not only can you enhance your projects, but you can also contribute to the Django community, helping fellow developers along the way.
"The greatest value can emerge when we extend not just our skills, but the capabilities of what we build together."
to fully exploit what Django can offer.
Best Practices for Django Development
When diving into Django development, adhering to certain best practices can set the stage for long-term project success and maintainability. This aspect isn't just about adhering to rules; it’s about creating a robust framework that allows for scalability and efficiency in your work. Maintaining high standards is not only beneficial for the initial development phase but can also save time and headaches down the road.
A cohesive approach to best practices can help streamline processes and ensure that developers create applications that are easier to understand, less prone to bugs, and that fit well within the Django ecosystem. Here are some key elements that one must consider:
- Consistency: Coding standards promote a consistent style, which makes it easier for different members of the team to read and understand each other's code.
- Maintainability: Adhering to established patterns makes it simpler to revisit or update code in the future, whether due to changing requirements or the onboarding of new developers.
- Performance Optimization: By following best practices, developers often find themselves using Django’s features more efficiently, leading to better application performance.
"Good code is its own best documentation."
– Stephen C. Johnson
Adhering to Coding Standards
Django is all about elegance and simplicity. So, maintaining clean and consistent coding standards is essential. These standards act as guidelines that dictate how to write code – naming conventions, spacing and indentation, and even how to structure files and directories.
For instance, following the PEP 8 style guide can help maintain clarity and uniformity throughout your project. Code readability is crucial; a well-structured codebase can mean the difference between a project that is a joy to work on and one that feels like pulling teeth.
Here are a few coding conventions to keep in mind:
- Use meaningful variable names that convey the purpose of the variable clearly.
- Keep line lengths to a maximum of 79 characters to ensure better readability.
- Employ docstrings for all public modules, functions, classes, and methods.
These practices promote a culture of clarity and efficiency, boosting your development experience immensely. Plus, when everyone on the team subscribes to the same standards, it really enhances collaboration.
Documentation and Code Maintainability
Documentation often takes a backseat in development, but it’s foundational for maintainability. Without adequate documentation, your Django project can quickly turn into a jumble of confusion. When it’s time to revisit your code after a few months (or years!), you might feel like you’re trying to decode ancient hieroglyphs.
A good rule of thumb is to document while you code. Including comments that clarify your thought process helps future developers (or your future self) understand the why behind specific choices.
To enhance maintainability:
- Document your API endpoints and models clearly. This helps anyone who might work with your codebase in the future.
- Utilize tools like Sphinx or MkDocs to automatically generate documentation from your comments and docstrings.
- Keep your README file updated. It should provide an overview of the project, how to set it up, and examples of how to use it.
In short, effective documentation is more than just a chore; it is an integral part of the development lifecycle. It allows developers to solve problems more quickly and reduces the risk of misunderstandings.
Embracing best practices, capturing clear coding standards, and committing to thorough documentation are cornerstones of effective Django development. Each of these elements contributes to not just a successful project, but a thriving development culture that values both efficiency and collaboration.
The Future of Django
As we pave the way into the landscape of modern development, understanding the future of Django holds significant importance. This web framework has consistently shown its adaptability and resilience, ensuring it remains at the forefront of web application development. Here, we will explore the trends shaping Django, its pivotal role in emerging technologies, and the essential considerations for developers and users alike.
Evaluating Trends in Web Development
In today’s fast-paced environment, web development trends are akin to the tide; they swirl in and out often. It’s crucial for those wielding Django to stay on the pulse of what’s happening in the broader web ecosystem. Below are several current trends:
- Microservices Architecture: Rather than monolithic structures, developers are tilting toward breaking applications into smaller, manageable pieces. Django facilitates this with its modular approach. Splitting applications into distinct components improves scalability and manageability.
- Serverless Computing: The rise of serverless architectures allows developers to focus more on writing code rather than managing infrastructure. Django aligns well with such models, enabling developers to deploy applications quickly and efficiently.
- Single Page Applications (SPAs): The demand for responsive and interactive applications continually rises. Django works hand-in-hand with JavaScript frameworks like React and Angular to create SPAs that enhance user experience.
"Staying aware of trends is like taking the pulse of the tech world; missed beats can lead to outdated practices."
These trends signal that Django is positioned to embrace the future, but recognizing and adapting to them is essential for success.
Django's Role in Emerging Technologies
Emerging technologies are evolving rapidly, and Django is not left behind. Its role in the following areas showcases its versatility and forward-thinking capabilities:
- Artificial Intelligence and Machine Learning: Integrating AI capabilities into applications is becoming commonplace. Django’s flexibility allows it to be the backbone of applications harnessing these technologies. One can easily develop RESTful APIs that serve machine learning models.
- IoT (Internet of Things): As smart devices proliferate, Django’s robustness can cater to extensive IoT applications. It can handle multiple requests from various devices, becoming the perfect mediator between hardware and users.
- Cloud Computing: Django’s seamless integration with cloud services supports dynamic application hosting. Developers can leverage platforms like Amazon Web Services or Microsoft Azure, enhancing deployment and scalability.
In summary, as these technologies evolve, Django’s capacity for adaptation will dictate its relevance. Developers need to remain vigilant, continuously learning and integrating these innovations to ensure they are utilizing Django's full potential.
By embracing the future, Django doesn't merely strive to keep up; it aims to lead, offering developers the tools they need to navigate the complexities of tomorrow's web landscapes.
End and Further Learning Resources
In the final stretch of exploring Django recipes, it’s essential to reflect on what we have learned and where to go from here. The conclusion of any comprehensive guide serves as a pivotal moment to tie loose ends together and solidify understanding. An insightful summary helps one grasp the overall journey through Django’s landscape. Not only does it recap key points, but it also points to the ongoing nature of learning and technology.
The benefits of wrapping up this guide effectively cannot be understated. For any housewife looking to make sense of vast information like this, a well-summary is like a map after a long journey— it reveals locations of landmarks that might have been overlooked along the way. A suitable conclusion can also encourage individuals to embark on their next steps with confidence, utilizing the practices learned to foster real-life applications.
Additional resources for further learning should become indispensable companions at this stage. After all, technology never stops evolving, and neither should our quest for knowledge. Whether it’s online courses or must-read books, they not only fill the gaps in our understanding but also refine our skills. They foster a practical approach to concepts and techniques introduced in this guide.
"Education is not the filling of a pail, but the lighting of a fire." – William Butler Yeats
Summarizing Key Takeaways
Throughout this guide, several vital takeaways have emerged:
- Django's Core Principles: Understanding models, views, and templates is fundamental for creating robust applications.
- Best Practices: Adhering to coding standards and maintaining documentation leads to better maintainability and scale.
- Real-time Applications: The ability to develop RESTful APIs shows Django's adaptability within modern web landscapes.
- Testing and Debugging: Building a solid habit of testing ensures smoother deployments and high-quality applications.
- Extending Functionality: Knowledge of middleware and signals effectively enhances what you can do with Django.
Recommended Books and Online Courses
Furthering your understanding of Django can take many forms. Here’s a selection of recommended reading materials and courses that can act as your guides:
- Books:
- Online Courses:
- Community Resources:
- Django for Beginners by William S. Vincent – A simple, clear walkthrough for newcomers.
- Two Scoops of Django by Daniel and Audrey Roy Greenfeld – A deeper dive into best practices that every Django developer should know.
- Django for Everybody Specialization on Coursera – Ideal for those who want to gain hands-on experience.
- Django REST Framework by Udemy – A comprehensive course focusing on API development using Django.
- Django Reddit Page – A space to share news, challenges, and best practices within the Django community.
- Django Documentation – Dive into the official site for up-to-date info and guides.
With these resources in hand, you'll ensure that your journey with Django is just beginning. The world of web development is vast and continuously evolving, and having reliable resources will enable you to stay ahead of the curve and master your own Django recipes effectively.