Mastering CMake: Streamline Your Build Process
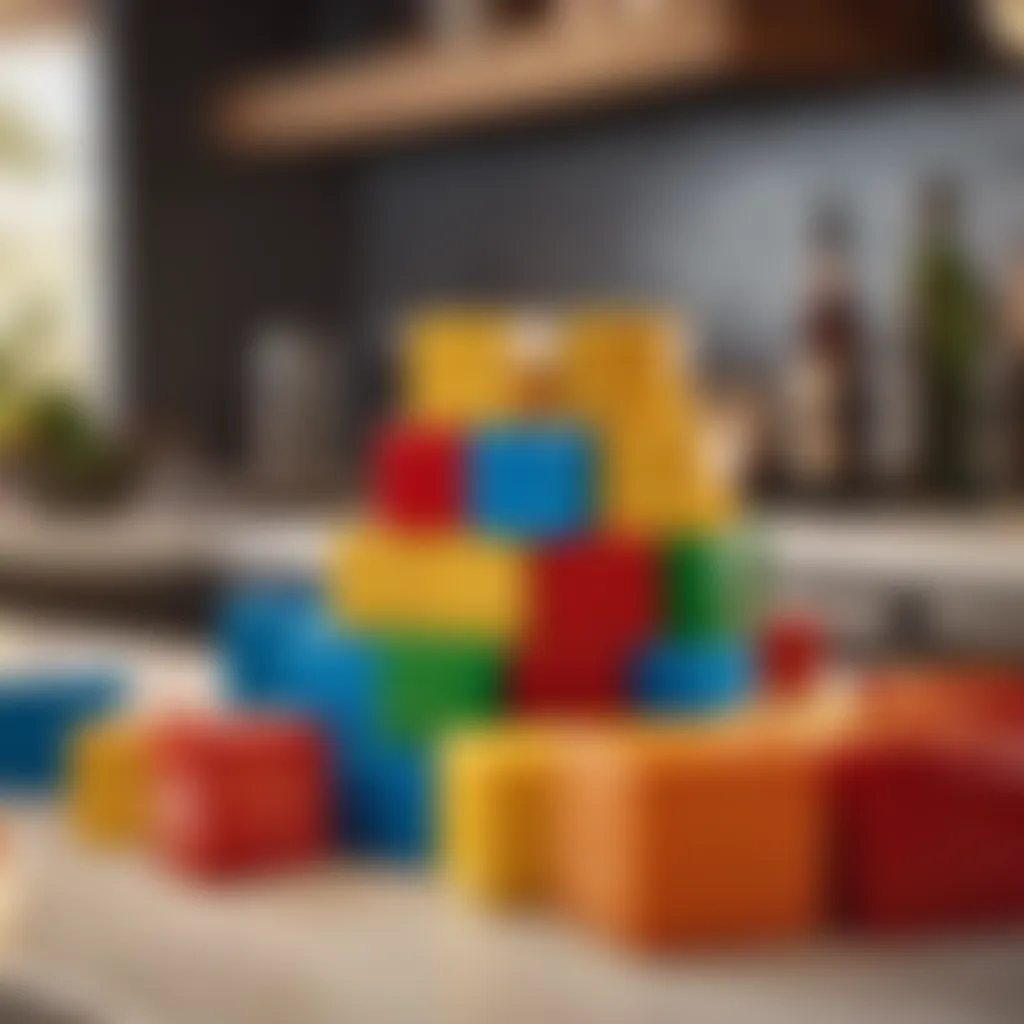
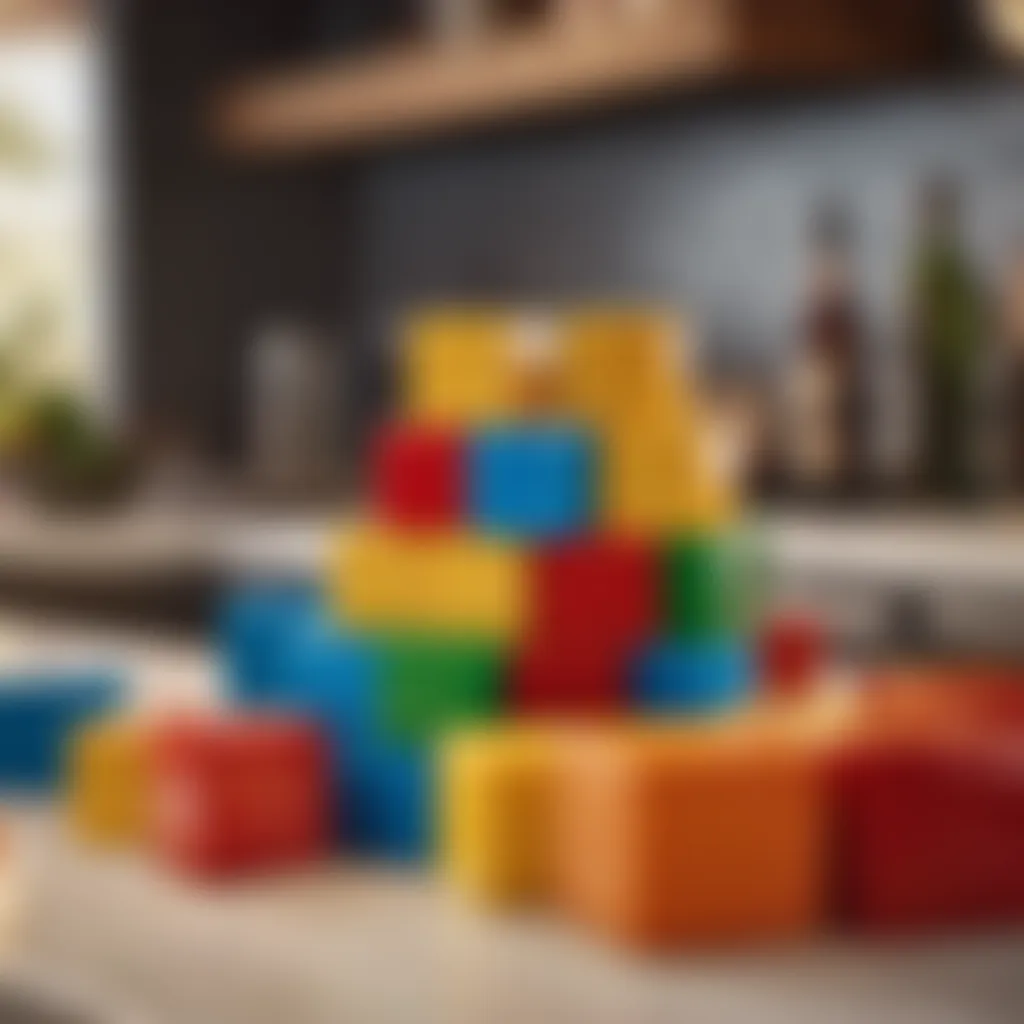
Intro
In the ever-evolving world of software development, efficiency can be the shining star that lights the way through the often complex landscape of project build systems. Among the many tools designed to simplify this journey, CMake stands out as a robust solution for developers seeking to streamline their build processes. Think of it as your secret ingredient—vital, versatile, and indispensable.
Whether you're new to the realm of build systems or a seasoned coder, understanding CMake is a must if you want your projects to run smoothly and efficiently. This recipe outlines everything you need to expertly navigate through CMake's capabilities, equipping you with the skills to manage dependencies, configure projects, and apply best practices. With clear steps and illustrative examples, you'll be ready to tackle any build-related challenge that comes your way.
Let's jump into the details that will not only clarify CMake's functionality but also enhance your productivity in software development.
Intro to CMake
Understanding CMake is pivotal as it lays the groundwork for developers aiming to smooth out the complexities of software build processes. This section is where we set the stage, shedding light on what CMake is and why it's a critical component in today’s development environment. It's like preparing all ingredients before cooking—everything you need must be lined up and ready to go.
What is CMake?
CMake is essentially a build system generator. It doesn’t compile any code on its own but instead generates the files needed for a specific environment so that various compilers can do the actual building. Think of it as a translator for your source code and the different environments it’s meant to thrive in. The beauty of CMake lies in its versatility; it can adapt to various compilers and platforms. This makes it a darling among developers who want their projects to be portable, be it on Windows, macOS or Linux.
The Evolution of Build Systems
Over the years, build systems have evolved from being straightforward, single-platform compilers to sophisticated tools capable of handling multiple platforms and dependencies. Initially, developers relied heavily on manual makefiles, which were tedious and challenging to maintain. As projects grew in size and complexity, the need for a more streamlined approach became evident.
CMake emerged to fill this gap, allowing for greater flexibility and efficiency. It handles complicated dependencies and can create build files for several compilers with just one configuration file. This evolution represents a fundamental shift in how developers approach the build process, minimizing errors and saving time.
Why Use CMake?
There are numerous reasons to adopt CMake as part of your development toolkit:
- Cross-Platform Compatibility: CMake generates build instructions for various platforms, making it the Swiss Army knife of build tools. You can craft a project on one system and build it seamlessly on another.
- Dependency Management: It takes the headache out of managing libraries and other dependencies, ensuring that everything fits together like pieces of a jigsaw puzzle.
- Community Support: Given its widespread use, there's a treasure trove of resources, forums, and documentation available. If you get stuck, chances are someone else has been there before you.
In summary, CMake plays an essential role in modern software development. From understanding its core functions to appreciating its evolution and benefits, this part sets the base for what’s to come. Armed with this knowledge, readers will be better positioned to tackle their build processes with CMake efficiently.
Getting Started with CMake
Getting to grips with CMake is like setting the stage before the play begins. Without this step, all the rehearsals in the world won't get your show off the ground. Emphasizing the importance of starting with CMake lays the foundation for smoother software development. First, you need to install it correctly, then establish a coherent directory structure, and finally configure your project for it to work seamlessly. In this section, we’ll cover the essentials that lay the groundwork for successful project builds.
Installation Process
Before any cooking begins, gathering the right ingredients is essential. The same can be said for installing CMake. It's slighty straightforward, but here’s a brief layout of how to go about it:
- Get the Right Version: Visit the CMake website and grab the version suited for your operating system. Whether you're on Windows, macOS, or Linux, the options are clear as day.
- Follow the Steps: Installation procedures differ slightly by OS, so follow the specific instructions:
- Verify the Installation: Once installed, open your terminal and run . If it returns the version, you are set to move ahead.
- On Windows, you might find a .msi installer handy; just click through the prompts and you’re golden.
- For macOS, consider using Homebrew with the command .
- If you are a Linux aficionado, the package manager command will depend on your distribution, such as for Ubuntu.
Basic Project Structure
Now that you have CMake working, it’s time to whip up a solid project structure. Think of this as organizing your kitchen before cooking – it makes everything easier. A well-structured project enhances clarity and efficiency:
- Root Directory: The starting point of your project. This is where your CMakeLists.txt file will live.
- Source Folders: Create directories for source code, often named , where main code files are housed.
- Header Files: Keep your header files organized, typically in a folder, which helps in managing interfaces well.
- Build Directory: A separate directory for build outputs keeps your workspace neat and tidy. Typically named , this is where CMake will generate the necessary files during the build process.
- Example Structure:
Adopting this structure will prevent a headache down the line, especially in larger projects.
Configuring Your First CMake Project
With the structure in place, the next step is to start configuring. It’s like mixing up your ingredients just right:
- Create CMakeLists.txt: This is the heart of your project. Here’s a simple configuration outline:
Specify the ++ standard version
set(CMAKE_CXX_STANDARD 14)
Add source files
add_executable(MyExecutable src/main.cpp)
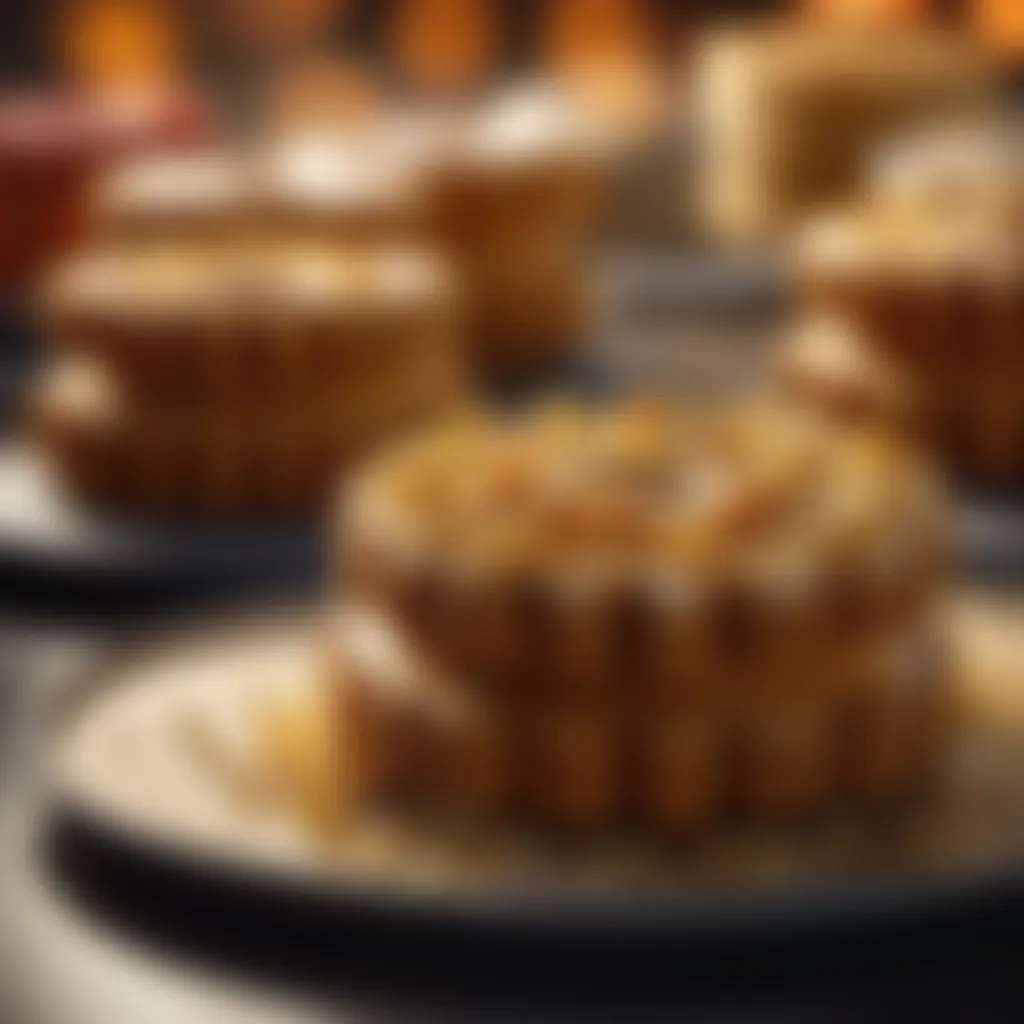
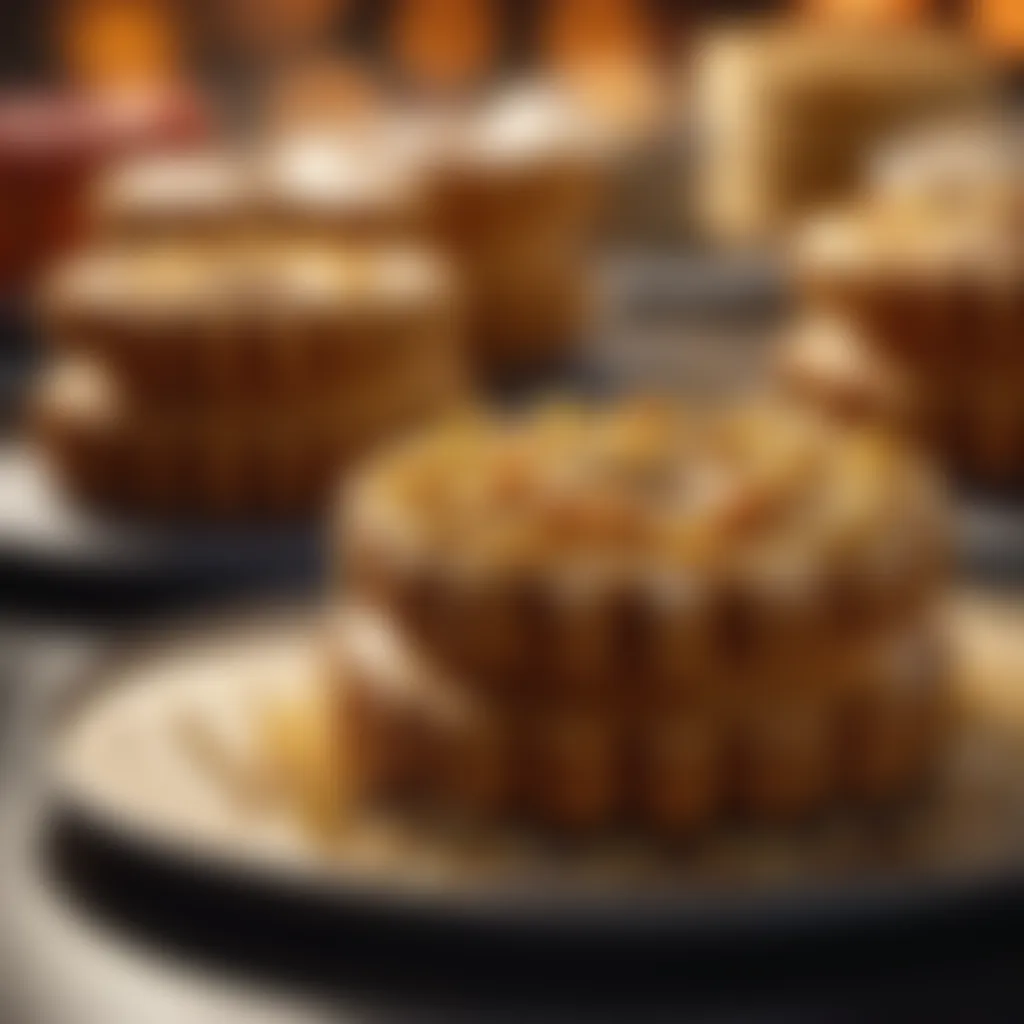
- Invoke CMake: Run from within the directory. CMake will process the instructions in your CMakeLists.txt.
- Build the Project: Use the command (or ), and watch it all come together. If successful, your executable will be created, ready for testing.
Remember: Each project is unique. Don't shy away from tweaking these steps to fit your particular scenarios.
Getting started with CMake might seem like an uphill battle at first, but with an organized approach, you'll find the tools in your hands to streamline the building process effectively. Keep experimenting and refining, and soon enough, you will find your rhythm!
Core Concepts of CMake
Understanding the core concepts of CMake is essential for anyone looking to navigate the intricacies of software build processes effectively. These fundamental ideas lay the groundwork for creating a seamless workflow. Without grasping these concepts, developers might find themselves mired in confusion when trying to configure their projects.
By investing the time to learn about these core concepts, you will be better equipped to manage your build effectively. Notably, this insight into CMake can lead to substantial improvements in project organization, quicker build times, and the ability to handle intricate projects without the usual headaches often associated with dependencies and configurations.
CMakeLists.txt Explained
The file is where the CMake magic begins. This script acts like a recipe card, detailing the ingredients and steps needed for your project build. Imagine your favorite recipe but for software development – it describes how to compile the code, link libraries, and set various configurations.
At its core, a file contains command directives which tell CMake what to do. A simple example would look like this:
In this snippet, specifies the minimum version of CMake required to process the file. The command names your project. Finally, the command tells CMake to compile an executable named from the source file .
You need to be mindful of how you structure this file. A chaotic might turn into a real nightmare, yielding errors that can be hard to track down. Having clear sections for each part of your project not only helps CMake understand your intentions but also aids human readability, which can be crucial when collaborating with other developers.
Understanding Variables and Functions
CMake encourages the use of variables and functions to streamline the build process, making managing configurations much easier. Just like cooking with different ingredients, variables store values – be it paths, options, or flags – that you might want to reuse in various parts of your project. This capability allows you to maintain flexibility and reduce redundancy in your configuration.
An example of setting a variable would be:
In this example, the variable is set to your include path, and later referenced in the command. This not only makes your script cleaner but also allows easy adjustments should the include path change.
Functions further enhance this concept by grouping a set of commands that can be executed multiple times throughout your . This is akin to having prepared sauces in a kitchen; measure it once and use it wherever needed.
Targets: An Overview
Targets are the building blocks of CMake. They represent outputs of the build process. Think of a target as a dish you intend to serve: it could be an executable, a library, or even a custom command. Each target encapsulates its specific behaviors and settings.
Creating a target typically involves the for executables or for libraries. For instance:
Here, is a static library that CMake will compile from . Understanding how to manage targets is pivotal because it allows you to specify dependencies. If your target needs another, you can tell CMake.
For instance, if depends on , you would link them as follows:
In summary, grasping these core concepts is critical. The ability to effectively manage , harness variables and functions, and configure targets means that developers will spend less time wrestling with their build system and more time focusing on coding.
"A well-structured CMake system can be the linchpin for successful software development, kind of like a well-organized kitchen in a bustling restaurant."
Familiarizing yourself with these ideas will pave the way to optimizing your own build processes and boosting your productivity.
Advanced CMake Techniques
As software development evolves, so too does the complexity of projects, leading to a rising demand for effective build tools like CMake. Advanced techniques represent a crucial juncture in any developer's journey, transforming how they manage dependencies and structure commands. In this section, we dive into three key areas: handling dependencies, creating custom commands, and using CMake for testing. Mastering these elements not only streamlines workflow but also enhances reliability and adaptability in build processes.
Handling Dependencies
Dependencies in software development are like ingredients in a recipe; the right ones, in the right proportions, will yield a successful dish. CMake's ability to manage these dependencies is indispensable. By utilizing the function, developers can easily incorporate external libraries, ensuring that their projects have the necessary components to run smoothly.
This part of CMake also allows for fine-grained control over how dependencies are linked. You might have used a library that’s specifically tailored for your project, but if it’s not accounted for in CMake, your build might just stumble in the dark. Here's a practical illustration:
With this setup, when building your project, CMake is aware of and will correctly link it to . Without such declarations, developers may encounter errors that not only frustrate but can derail timelines.
Creating Custom Commands
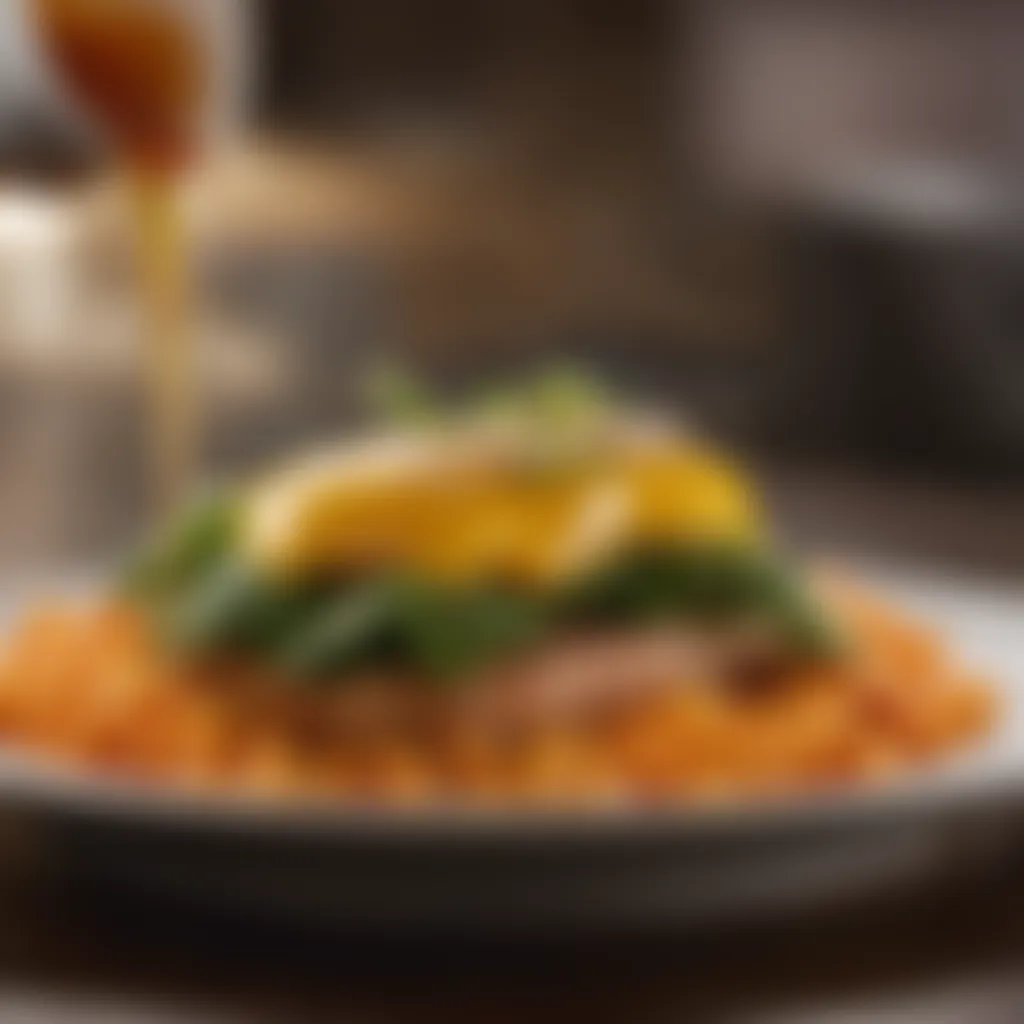
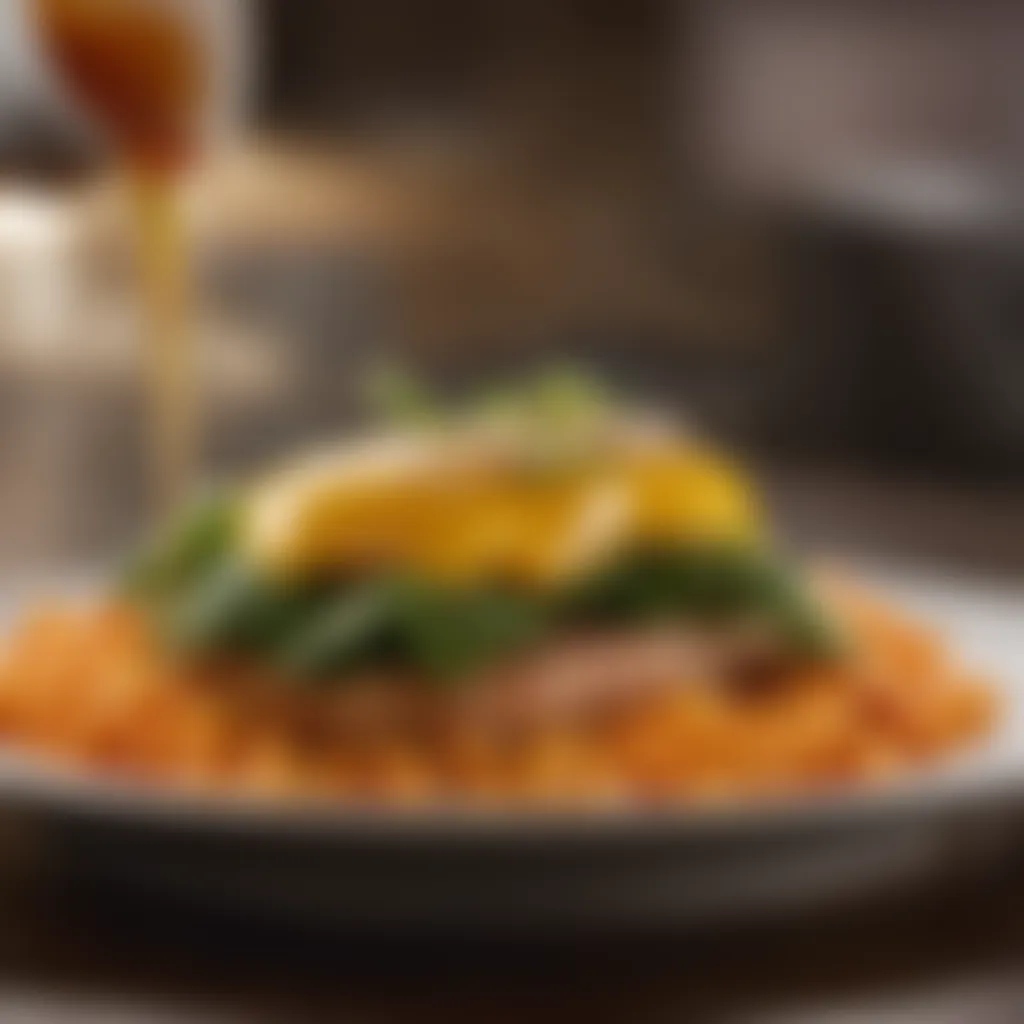
Custom commands in CMake act like secret spices, offering a unique flair to your build processes. Sometimes, you need to execute specific tasks, like generating files or performing actions before or after the build. CMake makes this out as simple as pie with commands like and .
For instance, if your project involves generating documentation or assets that are necessary before the main build process, you can define:
This setup guarantees that every time you compile your project, is created from automatically, streamlining the process.
Using CMake for Testing
Testing is an integral part of software development, and CMake simplifies this process with built-in support. By incorporating testing commands, you lay down the vital groundwork for code reliability. The and functions allow you to define test cases just as if you were adding regular files to your project.
When you execute tests using CMake's integrated testing framework, such as CTest, you'll improve your code’s robustness. Here's a quick code snippet for reference:
This simple command ensures that your testing suite runs alongside the regular build. When everything's set up correctly, and you've ensured dependencies are managed, you’ll catch any hiccups before going live.
In summary, mastering these CMake advanced techniques not only simplifies your build process but helps forge a mature development workflow that can adapt to the ever-changing landscape of software engineering. As you become comfortable with these concepts, you'll find that developing complex applications becomes a more intuitive and efficient experience.
CMake Best Practices
When it comes to employing CMake for building software, adhering to best practices can significantly improve the maintainability, readability, and efficiency of your projects. Good habits in CMake not only enhance performance but also save time and trouble down the line. This segment explores critical aspects of CMake best practices, breakdowns of key elements, and the considerations that developers should take into account.
Organizing CMakeLists files
A well-organized CMakeLists.txt file can be the difference between a smooth development experience and one fraught with confusion. Start by using a clear hierarchy in your project. Neatly organizing files into directories and having a CMakeLists.txt file in each of these directories streamlines the build process. Let’s start with a few pointers:
- Parent and Child CMakeLists: It’s instrumental to have a parent CMakeLists.txt in your root directory that can configure global settings or variables. Each subdirectory should have its own file that handles specific targets or modules.
- Structure: Present a clear structure to easily navigate. Aim to group files logically, enabling quick access to the relevant segments when needed. A common approach is to have a folder for source files and a folder for headers, further divided into modules or functionalities.
- Comments for Clarity: Do not shy away from using comments. Writing explanations for complex sections of your files not only helps others but serves as a good reminder for yourself when looking back later.
Adopting this structured approach makes it easier to identify where changes are needed, avoiding pitfalls that come from a network of tangled dependencies or misplaced settings.
Version Control Considerations
Utilizing version control with CMake makes managing changes simpler and more effective. Here are considerations to keep in mind:
- Ignore Build Files: It’s prudent to avoid tracking build directories in your version control system. These can often bloat your repository with files generated during building. Using a file can manage this effectively.
- Keep Configurations Clear: Maintain clear documentation within your repository. If different branches require distinct CMake configuration settings, clearly state this in README files or related documentation.
- Using Tags: Tagging releases is essential for tracking changes over time. It allows you to easily return to a previous state of your project when needed. This is especially relevant when working alongside other contributors or when easing transitions between your development and production environments.
Effective version control not only keeps your project tidy but also mitigates the stress of collaborating in larger teams, ensuring that changes are easily traceable and reversible.
Cross-Platform Compatibility
In today's diverse environment, your code can end up running on various platforms. CMake shines in its ability to inherently support cross-platform compatibility. Pay special attention to these strategies:
- Abstract Dependencies: Define your dependencies based on library checks, avoiding platform-specific code unless necessary. The command can often create a reliable foundation.
- Conditional Logic: Use platform checks to tailor configurations. Basic configurations may leverage commands like or to handle specifics for different operating systems. Yet, be careful; go overboard with conditionals, and you may end up with a CMakeLists file that's hard to read.
- CMake System Variables: Utilize CMake's built-in variables like which can provide information about the target system. This helps you create a smoother, platform-agnostic build process.
By putting an emphasis on cross-platform capabilities, you create a more accessible code base, allowing other developers or users comfortable in their own environments to benefit from your work.
Remember, adhering to best practices might seem tedious initially, but the smooth sailing experience when building, maintaining and sharing your projects is worth the effort!
Troubleshooting Common Issues
Understanding how to troubleshoot common issues in CMake is akin to having a roadmap whenever you hit a bump in the road. Facing errors in the build process can be frustrating, but knowing how to tackle these problems can make all the difference. This section seeks to empower you with the knowledge to identify, analyze, and ultimately resolve issues, ensuring your journey through CMake remains smooth and productive.
CMake, while powerful, is not without its hiccups. Incorrect configurations, missing dependencies, or even simple typos can lead to a myriad of errors. Recognizing these potential pitfalls means less time pulling your hair out and more time focusing on creating excellent software. It’s vital to have a toolkit of common errors and fixes at your disposal, as well as strategies to debug CMake builds effectively.
Common Errors and Fixes
CMake has a reputation for throwing its fair share of curveballs, particularly when it comes to syntax and environment setups. Some of the most frequent issues include:
- Syntax Errors: Missing a semi-colon or using the wrong variable syntax can cause the build process to fail.
- Path Issues: If CMake can’t find the necessary files, it will halt, leading to unwanted delays. Always double-check your paths.
- Dependency Problems: Some libraries might not be installed correctly, leading to missing dependencies. Ensuring libraries are properly linked is vital.
- Version Conflicts: Using an outdated version of CMake with newer projects can result in compatibility issues.
To address these problems, the following approaches can help:
- Review Error Messages: Don’t overlook the error messages. They usually provide clues about what went wrong.
- Checking CMakeLists.txt: Go through your CMakeLists.txt file line by line. Missing or misconfigured instructions are often the culprit.
- Utilize CMake's Verbose Mode: Running provides insight into what CMake is processing, helping you pinpoint where things go awry.
In case you encounter persistent errors, always ensure to consult the CMake documentation. It hosts a wealth of community-driven solutions to many of the common problems that might stymie new users.
Debugging CMake Builds
When it comes to debugging, patience is key. CMake builds can misbehave for several reasons, and pinpointing the root cause often requires a systematic approach. Here are strategies to help guide you:
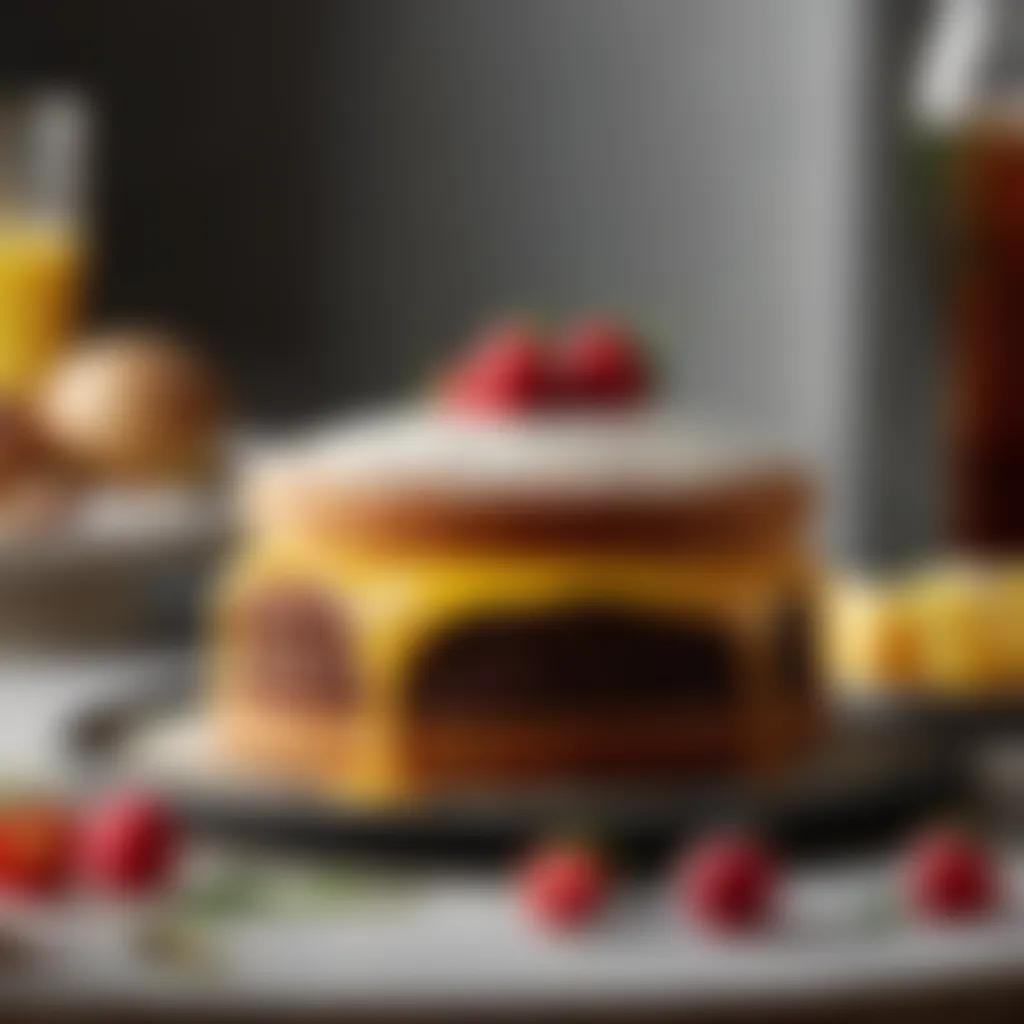
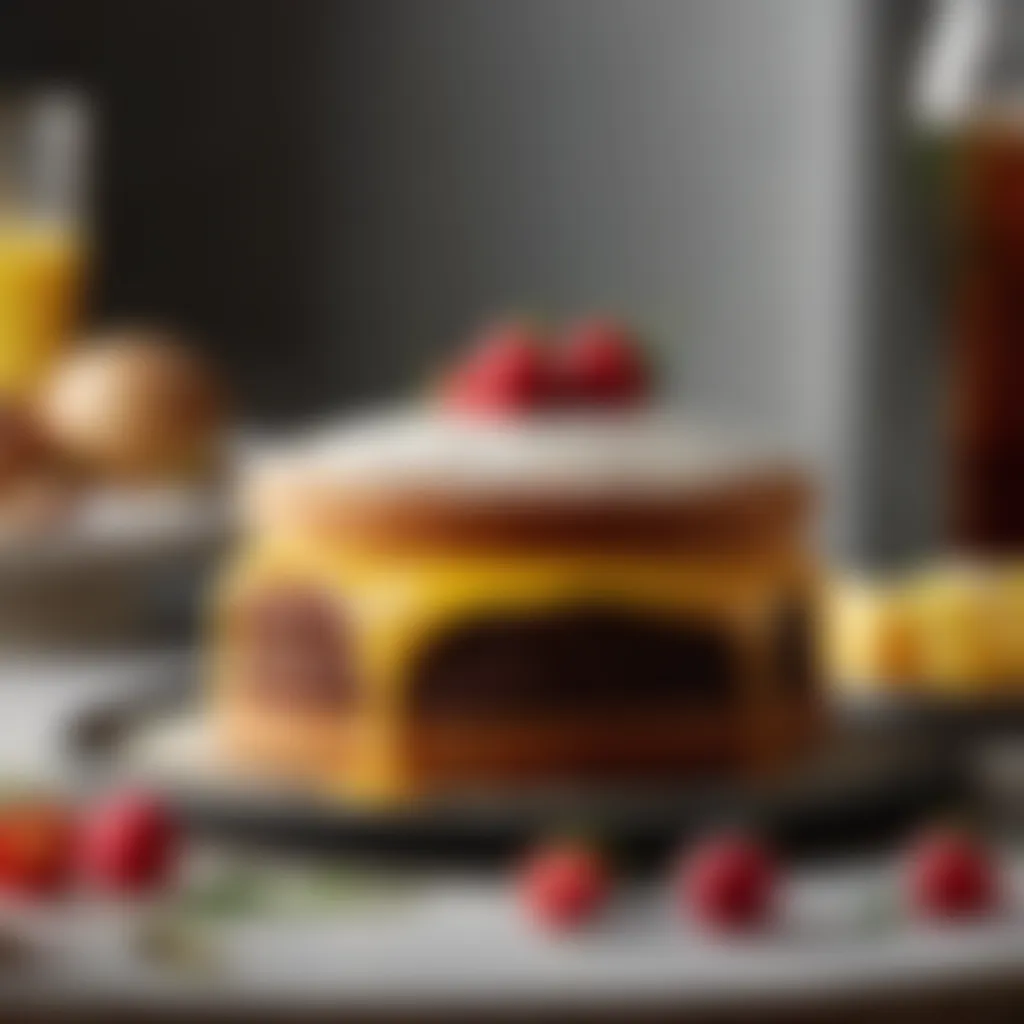
- Use Built-In CMake Tools: CMake provides various debugging tools such as and . These can be beneficial for altering and inspecting build parameters on the fly.
- Verbose Output: Run the build commands with additional verbosity using in Unix-like environments. This will surface detailed information about every step of the compile process.
- Incremental Build Approach: Rather than re-running the entire build after a change, try increments. This allows you to isolate changes and better understand their impact on the build.
- Integration with IDEs: Many Integrated Development Environments, like CLion or Visual Studio, offer better debugging options for CMake builds. They may highlight the issues directly in your code editor.
To summarise, debugging CMake builds doesn’t have to be a monumental task. With clear-headed troubleshooting, common errors can become manageable challenges. Knowing these aspects will transform your CMake usage from a perplexing puzzle into a well-defined process, enhancing not just productivity but also your coding experience.
Key Takeaway: Equipped with the right knowledge and tools, troubleshooting CMake can turn from a tedious task into an opportunity to learn and grow in your development journey.
Integrating CMake with Other Tools
Integrating CMake with other tools can really boost productivity and streamline development workflows. In a rapidly evolving tech world, working with software that plays nice together is indispensable. CMake shines bright as a versatile build manager, but its full potential often emerges only when paired with various systems and platforms.
For instance, most developers depend on Integrated Development Environments (IDEs) to simplify code editing, debugging, and project organization. CMake’s ability to integrate seamlessly with popular IDEs such as Visual Studio, CLion, and Eclipse is a game changer. This cooperation means that you can harness the debugging and visualization features of these IDEs without changing your project structure significantly. It allows for a smoother coding experience, enabling developers to devote more time to writing quality code instead of wrestling with configurations.
Additionally, leveraging Continuous Integration (CI) systems is crucial for maintaining code quality. When teams ensure that their builds are regularly tested and verified, the risk of introducing bugs into production decreases substantially. CMake plays a vital role in this setup as it can easily generate build scripts tailored for CI tools like Jenkins, Travis CI, or GitHub Actions. By automating the build process, teams can focus on innovation rather than manual setups, leading to enhanced project efficiency.
In summary, integrating CMake with other tools enhances the overall development experience. The amalgamation of CMake with IDEs and CI systems helps in creating more standardized and efficient workflows which, in turn, clears the path for quality software.
CMake with IDEs
Using CMake with IDEs provides a streamlined development process. When CMake is used to set up a project, it generates native build files for a variety of IDEs. This inherent compatibility means that once a developer configures their CMake setup, they can directly open their project in their IDE of choice. The beauty of this approach lies in that it allows developers to work within a familiar environment while still leveraging CMake's powerful capabilities.
- Visual Studio: With intuitive project setup and debugging tools, integrating CMake with Visual Studio makes complex builds easier. You can manage CMake settings within the IDE and use features like IntelliSense to improve coding efficiency.
- CLion: This JetBrains IDE is tailored for C++ and excels in handling CMake. Developers can enjoy seamless debugging and refactoring capabilities, thanks to its deep integration with CMake. Additionally, it automatically detects project changes and manages CMake cache intelligently.
- Eclipse CDT: CMake for Eclipse enables the use of complex C++ projects without a steep learning curve. Eclipse users can easily convert CMake files into Eclipse project files, ensuring that they have access to the extensive Eclipse ecosystem without the hassle.
Incorporating IDEs into the CMake framework allows developers to maintain a high level of productivity, reducing time spent on configuration and increasing focus on development tasks.
Continuous Integration Systems
CMake's compatibility with Continuous Integration systems is essential for modern development practices. Continuous Integration helps teams to build, test, and deploy their code more efficiently by automating these processes. Integrating CMake within CI systems ensures that each change made to the codebase is automatically tested, promoting a culture of quality and accountability in development.
By configuring CMake to work with CI tools, developers can easily run tests as part of their workflow. Popular choices for CI, like Jenkins and Travis CI, support CMake natively. When setting up a CI pipeline, CMake can create the necessary build environment and execute test suites, ensuring that everything is in working order before merging changes.
- Jenkins: With a pipeline set up, Jenkins can take CMake commands directly and compile your project whenever changes are pushed to the repository. Developers can customize build steps to fit their requirements, such as defining different build variants for development and production.
- Travis CI: For projects hosted on platforms like GitHub, Travis CI easily connects and runs CMake builds. Setting up a .travis.yml file allows developers to specify build environments and commands for testing, simplifying the CI process immensely.
Ultimately, integrating CMake with Continuous Integration systems not only automates builds and tests but also enhances collaboration within teams. As a result, developers spend less time troubleshooting and more time improving their software.
Future of CMake
The future of CMake stands as a beacon of possibility in the ever-evolving landscape of software development. As development teams across the globe strive for efficiency and versatility, CMake provides an adaptable framework that meets these needs head-on. Not only does it facilitate the management of complex projects, but it also keeps pace with modern trends in building software. Such an adaptability is paramount, considering that programming paradigms and technologies change rapidly. In this segment, we’ll explore the various aspects that shape the future of CMake, including emerging trends in build systems and the community that supports it.
Emerging Trends in Build Systems
In the fast-moving world of software development, trends have a way of shifting like sand beneath our feet. One significant trend that’s gaining traction is the rise of declarative build systems. Unlike imperative systems, which tell computers what to do step-by-step, declarative systems focus on specifying the desired outcome. CMake is moving towards this model, offering a more intuitive approach that can simplify the build process.
Furthermore, the integration of DevOps practices is reshaping how teams interact with build systems like CMake. With continuous integration and continuous delivery (CI/CD) on the rise, automating builds and tests is no longer a luxury but a necessity. CMake is keen to embrace this shift by providing tools that simplify integration with CI systems, thus enabling developers to spend less time troubleshooting and more time coding.
"Adopting emerging trends can keep your projects relevant and efficient in a landscape that's constantly shifting."
Additionally, the growing emphasis on cloud computing means that more teams are looking for cloud-native solutions. CMake is adapting accordingly, allowing developers to build projects that can be deployed seamlessly in cloud environments.
Community and Support Resources
A robust community can transform a good tool into a great one, and CMake is fortunate to have a dedicated following. The strength of this community is invaluable in shaping the future of CMake, offering support and resources that enhance user experience. From forums to documentation, these resources not only assist newcomers but also keep seasoned users informed of the latest developments.
Online platforms like Reddit and dedicated Facebook groups serve as informal hubs where users exchange ideas and solutions. These interactions often illuminate best practices and innovative uses of CMake that may not be covered in official documentation.
Moreover, comprehensive resources such as CMake’s official documentation are continuously updated to reflect new features and enhancements. As users tap into these materials, they can maximize their software projects' potential, blending tried-and-true methods with new techniques.
Finale
In wrapping up our exploration of CMake, it’s crucial to reflect on its essential role in the software build process. This article has delved into numerous facets of CMake, many of which play a significant part in boosting efficiency and productivity for developers. One of the main benefits of understanding the CMake system is the streamlining of project configuration through a series of intuitive steps and commands. The emphasis on core principles, advanced techniques, and best practices has painted a comprehensive picture of how CMake serves as a robust orchestrator in the development lifecycle.
Summary of Key Points
As we approach the end, let's summarize some of the pivotal points discussed throughout the article:
- CMake's Evolution: It has transitioned from a basic tool to a highly versatile build system compatible with various platforms and languages.
- Installing CMake: The straightforward installation process allows developers to begin leveraging its capabilities quickly.
- Effective Project Structures: An organized project structure can significantly ease the development process.
- CMakeLists.txt: Understanding this file is fundamental to utilizing CMake effectively.
- Dependency Management: Handling dependencies is simplified through clever CMake techniques, enhancing project stability.
This synthesis not only reinforces the material but also serves to highlight the scalable nature of CMake in accommodating diverse project needs.
Final Thoughts on CMake
When considering the future of build systems, CMake stands out as a frontrunner. Its adaptability, backed by a supportive community and a wealth of resources, places it in a unique position to continue evolving with emerging technologies. This flexibility ensures that whether you are working on a small application or a large-scale software project, CMake can grow alongside you.
Ultimately, mastering CMake offers developers a toolkit that not only facilitates smoother builds but also encourages a deeper understanding of the development process itself. Embracing this tool can lead to tangible improvements in project execution and overall software quality.
"A good build system is like a well-oiled machine; it operates in the background, yet its efficiency drives the overall project success."
By continually revisiting and refining one’s approach to CMake, you’ll find your building endeavors more systematic and less fraught with obstacles. It’s not merely about using a tool; it’s about integrating a philosophy of adaptability and efficiency into your workflow.